Start#
Week 1#
003 – Syntax And Your First App#
print("I love python")
I love python
print("i love programming")
i love programming
print(1)
print(2)
1
2
if True:
print(1)
1
006 – Some Data Types Overview#
type(10)
# all data in python is object
int
type(10.1)
float
type("hello")
str
type([1, 2, 3])
list
type((1, 2, 3))
tuple
print(type({"one": 1}))
<class 'dict'>
print(type(1 == 1))
<class 'bool'>
007 – Variables Part One#
# syntax => [variable name][assignment operator][value]
myVariable = "my value"
print(myVariable)
my value
my_value = "value"
print(my_value)
value
# print(name)
# name ="yay" will generate error
# must assign value first before printing
name = "baka" # single word
myName = "baka" # camelCase
my_name = "baka" # snake_case
008 – Variables Part Two#
Source Code : Original Code You Write it in Computer
Translation : Converting Source Code Into Machine Language
Compilation : Translate Code Before Run Time
Run-Time : Period App Take To Executing Commands
Interpreted : Code Translated On The Fly During Executionm
x = 10
x = "hello"
print(x) # dynamically typed language
hello
help("keywords") # reserved words
Here is a list of the Python keywords. Enter any keyword to get more help.
False class from or
None continue global pass
True def if raise
and del import return
as elif in try
assert else is while
async except lambda with
await finally nonlocal yield
break for not
a, b, c = 1, 2, 3
print(a, b, c)
1 2 3
009 – Escape Sequences Characters#
Escape Sequences Characters#
\b => Back Space
\newline => Escape New Line + \
\ => Escape Back Slash
‘ => Escape Single Quotes
” => Escape Double Quotes
\n => Line Feed
\r => Carriage Return
\t => Horizontal Tab
\xhh => Character Hex Value
print("hello\bword")
hellword
print("hello\
python")
hellopython
print("i love \\python")
i love \python
print("i love sinqle quote \"")
i love sinqle quote "
print("hello \nworld")
hello
world
print("123456\rabcd")
abcd56
print("hello \tworld")
hello world
print("\x4F\x73")
Os
010 – Concatenation And Trainings#
msg = "i love"
lang = "python"
print(msg + " "+lang)
i love python
full = msg + " " + lang
print(full)
i love python
a = "first\
second\
third"
b = "A\
B\
C"
print(a)
print(b)
first second third
A B C
print(a + "\n"+b)
first second third
A B C
print("hi"+1)
will produce an error
print(msg + " 1")
i love 1
Week 2#
011 – Strings#
myStringOne = "this is 'signle quote'"
print(myStringOne)
this is 'signle quote'
myStringTwo = "This is Double Quotes"
print(myStringTwo)
This is Double Quotes
myStringThree = 'This is Single Quote "Test"'
print(myStringThree)
This is Single Quote "Test"
myStringFour = "This is Double Quotes 'Test'"
print(myStringFour)
This is Double Quotes 'Test'
multi = """first
second
third
"""
print(multi)
first
second
third
test = '''
"First" second 'third'
'''
print(test)
"First" second 'third'
012 – Strings – Indexing and Slicing#
[1] All Data in Python is Object
[2] Object Contain Elements
[3] Every Element Has Its Own Index
[4] Python Use Zero Based Indexing ( Index Start From Zero )
[5] Use Square Brackets To Access Element
[6] Enable Accessing Parts Of Strings, Tuples or Lists
# index access single item
string = "i love python"
print(string[0])
print(string[-1])
print(string[9])
i
n
t
# slicing access multiple sequence items
# [start:end]
# [start:end:steps]
print(string[8:11])
print(string[:10])
print(string[5:10])
print(string[5])
print(string[:])
print(string[0::1])
print(string[::1])
print(string[::2])
yth
i love pyt
e pyt
e
i love python
i love python
i love python
ilv yhn
013 – Strings Methods Part 1#
a = " i love python "
print(len(a))
print(a.strip())
print(a.rstrip())
print(a.lstrip())
18
i love python
i love python
i love python
a = " hi "
print(a.strip())
hi
a = "#####hola#####"
print(a.strip("#"))
print(a.rstrip("#"))
print(a.lstrip("#"))
hola
#####hola
hola#####
b = "I Love 2d Graphics and 3g Technology and python"
print(b.title())
print(b.capitalize())
I Love 2D Graphics And 3G Technology And Python
I love 2d graphics and 3g technology and python
c, d, e = "1", "20", "3"
print(c.zfill(3))
print(d.zfill(3))
print(e.zfill(3))
001
020
003
g = "aHmed"
print(g.lower())
print(g.upper())
ahmed
AHMED
014 – Strings Methods Part 2#
a = "I love python"
print(a.split())
['I', 'love', 'python']
a = "I-love-python"
print(a.split("-"))
['I', 'love', 'python']
a = "I-love-python"
print(a.split("-", 1))
['I', 'love-python']
d = "I-love-python"
print(d.rsplit("-", 1))
['I-love', 'python']
e = "ahmed"
print(e.center(15))
print(e.center(15, "#"))
ahmed
#####ahmed#####
f = "I and me and ahmed"
print(f.count("and"))
print(f.count("and", 0, 10)) # start and end
2
1
g = "I love Python"
print(g.swapcase())
i LOVE pYTHON
print(g.startswith("i"))
print(g.startswith("I"))
print(g.startswith("l", 2, 12)) # start from second index
False
True
True
print(g.endswith("n"))
print(g.endswith("e", 2, 6))
True
True
015 – Strings Methods Part 3#
a = "I Love Python"
print(a.index("P")) # Index Number 7
print(a.index("P", 0, 10)) # Index Number 7
# print(a.index("P", 0, 5)) # Through Error
7
7
b = "I Love Python"
print(b.find("P")) # Index Number 7
print(b.find("P", 0, 10)) # Index Number 7
print(b.find("P", 0, 5)) # -1
7
7
-1
c = "ahmed"
print(c.rjust(10))
print(c.rjust(10, "#"))
ahmed
#####ahmed
d = "ahmed"
print(d.ljust(10))
print(d.ljust(10, "#"))
ahmed
ahmed#####
e = """First Line
Second Line
Third Line"""
print(e.splitlines())
['First Line', 'Second Line', 'Third Line']
f = "First Line\nSecond Line\nThird Line"
print(f.splitlines())
['First Line', 'Second Line', 'Third Line']
g = "Hello\tWorld\tI\tLove\tPython"
print(g.expandtabs(5))
Hello World I Love Python
one = "I Love Python And 3G"
two = "I Love Python And 3g"
print(one.istitle())
print(two.istitle())
True
False
three = " "
four = ""
print(three.isspace())
print(four.isspace())
True
False
five = 'i love python'
six = 'I Love Python'
print(five.islower())
print(six.islower())
True
False
# to check if i can use a name as a variable
seven = "osama_elzero"
eight = "OsamaElzero100"
nine = "Osama--Elzero100"
print(seven.isidentifier())
print(eight.isidentifier())
print(nine.isidentifier())
True
True
False
x = "AaaaaBbbbbb"
y = "AaaaaBbbbbb111"
print(x.isalpha())
print(y.isalpha())
True
False
u = "AaaaaBbbbbb"
z = "AaaaaBbbbbb111"
print(u.isalnum())
print(z.isalnum())
True
True
016 – Strings Methods Part 4#
# replace(Old Value, New Value, Count)
a = "Hello One Two Three One One"
print(a.replace("One", "1"))
print(a.replace("One", "1", 1))
print(a.replace("One", "1", 2))
Hello 1 Two Three 1 1
Hello 1 Two Three One One
Hello 1 Two Three 1 One
myList = ["Osama", "Mohamed", "Elsayed"]
print("-".join(myList))
print(" ".join(myList))
print(", ".join(myList))
print(type(", ".join(myList)))
Osama-Mohamed-Elsayed
Osama Mohamed Elsayed
Osama, Mohamed, Elsayed
<class 'str'>
017 – Strings Formatting Old Way#
name = "Osama"
age = 36
rank = 10
print("My Name is: " + name)
My Name is: Osama
print("My Name is: " + name + " and My Age is: " + age)
type error, cant concatenate string with int
print("My Name is: %s" % "Osama")
print("My Name is: %s" % name)
print("My Name is: %s and My Age is: %d" % (name, age))
print("My Name is: %s and My Age is: %d and My Rank is: %f" % (name, age, rank))
My Name is: Osama
My Name is: Osama
My Name is: Osama and My Age is: 36
My Name is: Osama and My Age is: 36 and My Rank is: 10.000000
n = "Osama"
l = "Python"
y = 10
print("My Name is %s Iam %s Developer With %d Years Exp" % (n, l, y))
My Name is Osama Iam Python Developer With 10 Years Exp
# control flow point number
myNumber = 10
print("My Number is: %d" % myNumber)
print("My Number is: %f" % myNumber)
print("My Number is: %.2f" % myNumber)
My Number is: 10
My Number is: 10.000000
My Number is: 10.00
# Truncate string
myLongString = "Hello People of Elzero Web School I Love You All"
print("Message is %s" % myLongString)
print("Message is %.5s" % myLongString)
Message is Hello People of Elzero Web School I Love You All
Message is Hello
018 – Strings Formatting New Way#
name = "Osama"
age = 36
rank = 10
print("My Name is: " + name)
My Name is: Osama
print("My Name is: {}".format("Osama"))
print("My Name is: {}".format(name))
print("My Name is: {} My Age: {}".format(name, age))
print("My Name is: {:s} Age: {:d} & Rank is: {:f}".format(name, age, rank))
My Name is: Osama
My Name is: Osama
My Name is: Osama My Age: 36
My Name is: Osama Age: 36 & Rank is: 10.000000
{:s} => String {:d} => Number {:f} => Float
n = "Osama"
l = "Python"
y = 10
print("My Name is {} Iam {} Developer With {:d} Years Exp".format(n, l, y))
My Name is Osama Iam Python Developer With 10 Years Exp
# Control Floating Point Number
myNumber = 10
print("My Number is: {:d}".format(myNumber))
print("My Number is: {:f}".format(myNumber))
print("My Number is: {:.2f}".format(myNumber))
My Number is: 10
My Number is: 10.000000
My Number is: 10.00
# Truncate String
myLongString = "Hello Peoples of Elzero Web School I Love You All"
print("Message is {}".format(myLongString))
print("Message is {:.5s}".format(myLongString))
print("Message is {:.13s}".format(myLongString))
Message is Hello Peoples of Elzero Web School I Love You All
Message is Hello
Message is Hello Peoples
# format money
myMoney = 500162350198
print("My Money in Bank Is: {:d}".format(myMoney))
print("My Money in Bank Is: {:_d}".format(myMoney))
print("My Money in Bank Is: {:,d}".format(myMoney))
My Money in Bank Is: 500162350198
My Money in Bank Is: 500_162_350_198
My Money in Bank Is: 500,162,350,198
{:&d}
will produce an error
# ReArrange Items
a, b, c = "One", "Two", "Three"
print("Hello {} {} {}".format(a, b, c))
print("Hello {1} {2} {0}".format(a, b, c))
print("Hello {2} {0} {1}".format(a, b, c))
Hello One Two Three
Hello Two Three One
Hello Three One Two
x, y, z = 10, 20, 30
print("Hello {} {} {}".format(x, y, z))
print("Hello {1:d} {2:d} {0:d}".format(x, y, z))
print("Hello {2:f} {0:f} {1:f}".format(x, y, z))
print("Hello {2:.2f} {0:.4f} {1:.5f}".format(x, y, z))
Hello 10 20 30
Hello 20 30 10
Hello 30.000000 10.000000 20.000000
Hello 30.00 10.0000 20.00000
# Format in Version 3.6+
myName = "Osama"
myAge = 36
print("My Name is : {myName} and My Age is : {myAge}")
print(f"My Name is : {myName} and My Age is : {myAge}")
My Name is : {myName} and My Age is : {myAge}
My Name is : Osama and My Age is : 36
Week 3#
019 – Numbers#
# Integer
print(type(1))
print(type(100))
print(type(10))
print(type(-10))
print(type(-110))
<class 'int'>
<class 'int'>
<class 'int'>
<class 'int'>
<class 'int'>
# Float
print(type(1.500))
print(type(100.99))
print(type(-10.99))
print(type(0.99))
print(type(-0.99))
<class 'float'>
<class 'float'>
<class 'float'>
<class 'float'>
<class 'float'>
# Complex
myComplexNumber = 5+6j
print(type(myComplexNumber))
print("Real Part Is: {}".format(myComplexNumber.real))
print("Imaginary Part Is: {}".format(myComplexNumber.imag))
<class 'complex'>
Real Part Is: 5.0
Imaginary Part Is: 6.0
[1] You Can Convert From Int To Float or Complex
[2] You Can Convert From Float To Int or Complex
[3] You Cannot Convert Complex To Any Type
print(100)
print(float(100))
print(complex(100))
100
100.0
(100+0j)
print(10.50)
print(int(10.50))
print(complex(10.50))
10.5
10
(10.5+0j)
print(10+9j)
# print(int(10+9j)) error
(10+9j)
020 – Arithmetic Operators#
[+] Addition
[-] Subtraction
[*] Multiplication
[/] Division
[%] Modulus
[**] Exponent
[//] Floor Division
# Addition
print(10 + 30)
print(-10 + 20)
print(1 + 2.66)
print(1.2 + 1.2)
40
10
3.66
2.4
# Subtraction
print(60 - 30)
print(-30 - 20)
print(-30 - -20)
print(5.66 - 3.44)
30
-50
-10
2.22
# Multiplication
print(10 * 3)
print(5 + 10 * 100)
print((5 + 10) * 100)
30
1005
1500
# Division
print(100 / 20)
print(int(100 / 20))
5.0
5
# Modulus
print(8 % 2)
print(9 % 2)
print(20 % 5)
print(22 % 5)
0
1
0
2
# Exponent
print(2 ** 5)
print(2 * 2 * 2 * 2 * 2)
print(5 ** 4)
print(5 * 5 * 5 * 5)
32
32
625
625
# Floor Division
print(100 // 20)
print(119 // 20)
print(120 // 20)
print(140 // 20)
print(142 // 20)
5
5
6
7
7
021 – Lists#
[1] List Items Are Enclosed in Square Brackets
[2] List Are Ordered, To Use Index To Access Item
[3] List Are Mutable => Add, Delete, Edit
[4] List Items Is Not Unique
[5] List Can Have Different Data Types
myAwesomeList = ["One", "Two", "One", 1, 100.5, True]
print(myAwesomeList)
print(myAwesomeList[1])
print(myAwesomeList[-1])
print(myAwesomeList[-3])
['One', 'Two', 'One', 1, 100.5, True]
Two
True
1
print(myAwesomeList[1:4])
print(myAwesomeList[:4])
print(myAwesomeList[1:])
['Two', 'One', 1]
['One', 'Two', 'One', 1]
['Two', 'One', 1, 100.5, True]
print(myAwesomeList[::1])
print(myAwesomeList[::2])
['One', 'Two', 'One', 1, 100.5, True]
['One', 'One', 100.5]
print(myAwesomeList)
myAwesomeList[1] = 2
myAwesomeList[-1] = False
print(myAwesomeList)
['One', 'Two', 'One', 1, 100.5, True]
['One', 2, 'One', 1, 100.5, False]
myAwesomeList[0:3] = []
print(myAwesomeList)
[1, 100.5, False]
myAwesomeList[0:2] = ["A", "B"]
print(myAwesomeList)
['A', 'B', False]
022 – Lists Methods Part 1#
myFriends = ["Osama", "Ahmed", "Sayed"]
myOldFriends = ["Haytham", "Samah", "Ali"]
print(myFriends)
print(myOldFriends)
['Osama', 'Ahmed', 'Sayed']
['Haytham', 'Samah', 'Ali']
myFriends.append("Alaa")
myFriends.append(100)
myFriends.append(150.200)
myFriends.append(True)
print(myFriends)
['Osama', 'Ahmed', 'Sayed', 'Alaa', 100, 150.2, True]
myFriends.append(myOldFriends)
print(myFriends)
['Osama', 'Ahmed', 'Sayed', 'Alaa', 100, 150.2, True, ['Haytham', 'Samah', 'Ali']]
print(myFriends[2])
print(myFriends[6])
print(myFriends[7])
Sayed
True
['Haytham', 'Samah', 'Ali']
print(myFriends[7][2])
Ali
a = [1, 2, 3, 4]
b = ["A", "B", "C"]
print(a)
[1, 2, 3, 4]
a.extend(b)
print(a)
[1, 2, 3, 4, 'A', 'B', 'C']
x = [1, 2, 3, 4, 5, "Osama", True, "Osama", "Osama"]
x.remove("Osama")
print(x)
[1, 2, 3, 4, 5, True, 'Osama', 'Osama']
y = [1, 2, 100, 120, -10, 17, 29]
y.sort()
print(y)
[-10, 1, 2, 17, 29, 100, 120]
y.sort(reverse=True)
print(y)
[120, 100, 29, 17, 2, 1, -10]
m = ["A", "Z", "C"]
m.sort()
print(m)
['A', 'C', 'Z']
Sort can’t sort a list that contains both of strings and numbers.
z = [10, 1, 9, 80, 100, "Osama", 100]
z.reverse()
print(z)
[100, 'Osama', 100, 80, 9, 1, 10]
023 – Lists Methods Part 2#
a = [1, 2, 3, 4]
a.clear()
print(a)
[]
b = [1, 2, 3, 4]
c = b.copy()
print(b)
print(c)
[1, 2, 3, 4]
[1, 2, 3, 4]
b.append(5)
print(b)
print(c)
[1, 2, 3, 4, 5]
[1, 2, 3, 4]
d = [1, 2, 3, 4, 3, 9, 10, 1, 2, 1]
print(d.count(1))
3
e = ["Osama", "Ahmed", "Sayed", "Ramy", "Ahmed", "Ramy"]
print(e.index("Ramy"))
3
f = [1, 2, 3, 4, 5, "A", "B"]
print(f)
f.insert(0, "Test")
f.insert(-1, "Test")
print(f)
[1, 2, 3, 4, 5, 'A', 'B']
['Test', 1, 2, 3, 4, 5, 'A', 'Test', 'B']
g = [1, 2, 3, 4, 5, "A", "B"]
print(g.pop(-3))
5
024 – Tuples Methods Part 1#
[1] Tuple Items Are Enclosed in Parentheses
[2] You Can Remove The Parentheses If You Want
[3] Tuple Are Ordered, To Use Index To Access Item
[4] Tuple Are Immutable => You Cant Add or Delete
[5] Tuple Items Is Not Unique
[6] Tuple Can Have Different Data Types
[7] Operators Used in Strings and Lists Available In Tuples
myAwesomeTupleOne = ("Osama", "Ahmed")
myAwesomeTupleTwo = "Osama", "Ahmed"
print(myAwesomeTupleOne)
print(myAwesomeTupleTwo)
('Osama', 'Ahmed')
('Osama', 'Ahmed')
print(type(myAwesomeTupleOne))
print(type(myAwesomeTupleTwo))
<class 'tuple'>
<class 'tuple'>
myAwesomeTupleThree = (1, 2, 3, 4, 5)
print(myAwesomeTupleThree[0])
print(myAwesomeTupleThree[-1])
print(myAwesomeTupleThree[-3])
1
5
3
# Tuple Assign Values
myAwesomeTupleFour = (1, 2, 3, 4, 5)
print(myAwesomeTupleFour)
(1, 2, 3, 4, 5)
myAwesomeTupleFour[2] = "Three"
print(myAwesomeTupleFour)
‘tuple’ object does not support item assignment
myAwesomeTupleFive = ("Osama", "Osama", 1, 2, 3, 100.5, True)
print(myAwesomeTupleFive[1])
print(myAwesomeTupleFive[-1])
Osama
True
025 – Tuples Methods Part 2#
myTuple1 = ("Osama",)
myTuple2 = "Osama",
print(myTuple1)
print(myTuple2)
('Osama',)
('Osama',)
print(type(myTuple1))
print(type(myTuple2))
<class 'tuple'>
<class 'tuple'>
print(len(myTuple1))
print(len(myTuple2))
1
1
a = (1, 2, 3, 4)
b = (5, 6)
c = a + b
d = a + ("A", "B", True) + b
print(c)
print(d)
(1, 2, 3, 4, 5, 6)
(1, 2, 3, 4, 'A', 'B', True, 5, 6)
myString = "Osama"
myList = [1, 2]
myTuple = ("A", "B")
print(myString * 6)
print(myList * 6)
print(myTuple * 6)
OsamaOsamaOsamaOsamaOsamaOsama
[1, 2, 1, 2, 1, 2, 1, 2, 1, 2, 1, 2]
('A', 'B', 'A', 'B', 'A', 'B', 'A', 'B', 'A', 'B', 'A', 'B')
a = (1, 3, 7, 8, 2, 6, 5, 8)
print(a.count(8))
2
b = (1, 3, 7, 8, 2, 6, 5)
print("The Position of Index Is: {:d}".format(b.index(7)))
print(f"The Position of Index Is: {b.index(7)}")
The Position of Index Is: 2
The Position of Index Is: 2
# Tuple Destruct
a = ("A", "B", 4, "C")
x, y, _, z = a
print(x)
print(y)
print(z)
A
B
C
Week 4#
026 – Set#
[1] Set Items Are Enclosed in Curly Braces
[2] Set Items Are Not Ordered And Not Indexed
[3] Set Indexing and Slicing Cant Be Done
[4] Set Has Only Immutable Data Types (Numbers, Strings, Tuples) List and Dict Are Not
[5] Set Items Is Unique
# Not Ordered And Not Indexed
mySetOne = {"Osama", "Ahmed", 100}
print(mySetOne)
{'Osama', 'Ahmed', 100}
print(mySetOne[0])
will produce an error.
Slicing Cant Be Done
mySetTwo = {1, 2, 3, 4, 5, 6}
print(mySetTwo[0:3])
Will also produce an error.
Has Only Immutable Data Types
mySetThree = {"Osama", 100, 100.5, True, [1, 2, 3]}
Error, unhashable type: ‘list’
mySetThree = {"Osama", 100, 100.5, True, (1, 2, 3)}
print(mySetThree)
{True, 'Osama', 100.5, 100, (1, 2, 3)}
# Items are Unique
mySetFour = {1, 2, "Osama", "One", "Osama", 1}
print(mySetFour)
{1, 2, 'One', 'Osama'}
027 – Set Methods Part 1#
a = {1, 2, 3}
a.clear()
print(a)
set()
b = {"One", "Two", "Three"}
c = {"1", "2", "3"}
x = {"Zero", "Cool"}
print(b | c)
print(b.union(c))
print(b.union(c, x))
{'2', 'One', '3', 'Two', 'Three', '1'}
{'2', 'One', '3', 'Two', 'Three', '1'}
{'2', 'One', '3', 'Two', 'Cool', 'Three', '1', 'Zero'}
d = {1, 2, 3, 4}
d.add(5)
d.add(6)
print(d)
{1, 2, 3, 4, 5, 6}
e = {1, 2, 3, 4}
f = e.copy()
print(e)
print(f)
e.add(6)
print(e)
print(f)
{1, 2, 3, 4}
{1, 2, 3, 4}
{1, 2, 3, 4, 6}
{1, 2, 3, 4}
g = {1, 2, 3, 4}
g.remove(1)
# g.remove(7) will remove an error
print(g)
{2, 3, 4}
h = {1, 2, 3, 4}
h.discard(1)
h.discard(7) # wont produce an error
print(h)
{2, 3, 4}
i = {"A", True, 1, 2, 3, 4, 5}
print(i.pop())
True
j = {1, 2, 3}
k = {1, "A", "B", 2}
j.update(['Html', "Css"])
j.update(k)
print(j)
{1, 2, 3, 'Html', 'B', 'A', 'Css'}
028 – Set Methods Part 2#
a = {1, 2, 3, 4}
b = {1, 2, 3, "Osama", "Ahmed"}
print(a)
print(a.difference(b)) # a - b
print(a)
{1, 2, 3, 4}
{4}
{1, 2, 3, 4}
c = {1, 2, 3, 4}
d = {1, 2, "Osama", "Ahmed"}
print(c)
c.difference_update(d) # c - d
print(c)
{1, 2, 3, 4}
{3, 4}
e = {1, 2, 3, 4, "X", "Osama"}
f = {"Osama", "X", 2}
print(e)
print(e.intersection(f)) # e & f
print(e)
{1, 2, 3, 4, 'X', 'Osama'}
{'Osama', 2, 'X'}
{1, 2, 3, 4, 'X', 'Osama'}
g = {1, 2, 3, 4, "X", "Osama"}
h = {"Osama", "X", 2}
print(g)
g.intersection_update(h) # g & h
print(g)
{1, 2, 3, 4, 'X', 'Osama'}
{'Osama', 2, 'X'}
i = {1, 2, 3, 4, 5}
j = {0, 3, 4, 5}
print(i)
print(i.symmetric_difference(j)) # i ^ j
print(i)
{1, 2, 3, 4, 5}
{0, 1, 2}
{1, 2, 3, 4, 5}
i = {1, 2, 3, 4, 5}
j = {0, 3, 4, 5}
print(i)
i.symmetric_difference_update(j) # i ^ j
print(i)
{1, 2, 3, 4, 5}
{0, 1, 2}
029 – Set Methods Part 3#
a = {1, 2, 3, 4}
b = {1, 2, 3}
c = {1, 2, 3, 4, 5}
print(a.issuperset(b))
print(a.issuperset(c))
True
False
d = {1, 2, 3, 4}
e = {1, 2, 3}
f = {1, 2, 3, 4, 5}
print(d.issubset(e))
print(d.issubset(f))
False
True
g = {1, 2, 3, 4}
h = {1, 2, 3}
i = {10, 11, 12}
print(g.isdisjoint(h))
print(g.isdisjoint(i))
False
True
030 – Dictionary#
[1] Dict Items Are Enclosed in Curly Braces
[2] Dict Items Are Contains Key : Value
[3] Dict Key Need To Be Immutable => (Number, String, Tuple) List Not Allowed
[4] Dict Value Can Have Any Data Types
[5] Dict Key Need To Be Unique
[6] Dict Is Not Ordered You Access Its Element With Key
user = {
"name": "Osama",
"age": 36,
"country": "Egypt",
"skills": ["Html", "Css", "JS"],
"rating": 10.5
}
print(user)
{'name': 'Osama', 'age': 36, 'country': 'Egypt', 'skills': ['Html', 'Css', 'JS'], 'rating': 10.5}
user = {
"name": "Osama",
"age": 36,
"country": "Egypt",
"skills": ["Html", "Css", "JS"],
"rating": 10.5,
"name": "Ahmed"
}
print(user)
{'name': 'Ahmed', 'age': 36, 'country': 'Egypt', 'skills': ['Html', 'Css', 'JS'], 'rating': 10.5}
Notice that it prints Ahmed not Osama as it is defined later.
print(user['country'])
print(user.get("country"))
Egypt
Egypt
print(user.keys())
dict_keys(['name', 'age', 'country', 'skills', 'rating'])
print(user.values())
dict_values(['Ahmed', 36, 'Egypt', ['Html', 'Css', 'JS'], 10.5])
languages = {
"One": {
"name": "Html",
"progress": "80%"
},
"Two": {
"name": "Css",
"progress": "90%"
},
"Three": {
"name": "Js",
"progress": "90%"
}
}
print(languages)
{'One': {'name': 'Html', 'progress': '80%'}, 'Two': {'name': 'Css', 'progress': '90%'}, 'Three': {'name': 'Js', 'progress': '90%'}}
print(languages['One'])
{'name': 'Html', 'progress': '80%'}
print(languages['Three']['name'])
Js
print(len(languages))
3
print(len(languages["Two"]))
2
frameworkOne = {
"name": "Vuejs",
"progress": "80%"
}
frameworkTwo = {
"name": "ReactJs",
"progress": "80%"
}
frameworkThree = {
"name": "Angular",
"progress": "80%"
}
allFramework = {
"one": frameworkOne,
"two": frameworkTwo,
"three": frameworkThree
}
print(allFramework)
{'one': {'name': 'Vuejs', 'progress': '80%'}, 'two': {'name': 'ReactJs', 'progress': '80%'}, 'three': {'name': 'Angular', 'progress': '80%'}}
031 – Dictionary Methods Part 1#
user = {
"name": "Osama"
}
print(user)
user.clear()
print(user)
{'name': 'Osama'}
{}
member = {
"name": "Osama"
}
print(member)
member["age"] = 36
print(member)
member.update({"country": "Egypt"})
print(member)
# Both ways are equivalent.
{'name': 'Osama'}
{'name': 'Osama', 'age': 36}
{'name': 'Osama', 'age': 36, 'country': 'Egypt'}
main = {
"name": "Osama"
}
b = main.copy()
print(b)
main.update({"skills": "Fighting"})
print(main)
print(b)
{'name': 'Osama'}
{'name': 'Osama', 'skills': 'Fighting'}
{'name': 'Osama'}
print(main.keys())
dict_keys(['name', 'skills'])
print(main.values())
dict_values(['Osama', 'Fighting'])
032 – Dictionary Methods Part 2#
user = {
"name": "Osama"
}
print(user)
user.setdefault("name", "Ahmed")
user.setdefault("age", 36)
print(user)
{'name': 'Osama'}
{'name': 'Osama', 'age': 36}
print(user.setdefault("name", "Ahmed"))
Osama
member = {
"name": "Osama",
"skill": "PS4"
}
print(member)
member.update({"age": 36})
print(member)
print(member.popitem())
print(member)
{'name': 'Osama', 'skill': 'PS4'}
{'name': 'Osama', 'skill': 'PS4', 'age': 36}
('age', 36)
{'name': 'Osama', 'skill': 'PS4'}
view = {
"name": "Osama",
"skill": "XBox"
}
allItems = view.items()
print(view)
{'name': 'Osama', 'skill': 'XBox'}
view["age"] = 36
print(view)
{'name': 'Osama', 'skill': 'XBox', 'age': 36}
print(allItems)
dict_items([('name', 'Osama'), ('skill', 'XBox'), ('age', 36)])
a = ('MyKeyOne', 'MyKeyTwo', 'MyKeyThree')
b = "X"
print(dict.fromkeys(a, b))
{'MyKeyOne': 'X', 'MyKeyTwo': 'X', 'MyKeyThree': 'X'}
user = {
"name": "Ahmed"
}
me = user
print(me)
{'name': 'Ahmed'}
user["age"] = 21
print(me)
{'name': 'Ahmed', 'age': 21}
Notice that
me
got updated becauseme
anduser
share the same data.
Week 5#
033 – Boolean#
[1] In Programming You Need to Known Your If Your Code Output is True Or False
[2] Boolean Values Are The Two Constant Objects False + True.
name = " "
print(name.isspace())
True
name = "Ahmed"
print(name.isspace())
False
print(100 > 200)
print(100 > 100)
print(100 > 90)
False
False
True
print(bool("Osama"))
print(bool(100))
print(bool(100.95))
print(bool(True))
print(bool([1, 2, 3, 4, 5]))
True
True
True
True
True
print(bool(0))
print(bool(""))
print(bool(''))
print(bool([]))
print(bool(False))
print(bool(()))
print(bool({}))
print(bool(None))
False
False
False
False
False
False
False
False
034 – Boolean Operators#
name = "ahmed"
age = 21
print(name == "ahmed" and age == 21)
True
print(name == "ahmed" and age > 21)
False
print(name == "ahmed" or age > 21)
True
print(name == "mohamed" or age > 21)
False
print(not age > 21)
True
print(not (name == "ahmed" and age > 21))
True
035 – Assignment Operators#
x = 10
y = 20
x = x+y
print(x)
30
A better way
a = 10
b = 20
a += b
print(a)
30
x = 30
y = 20
x = x-y
print(x)
10
a = 30
b = 20
a -= b
print(a)
10
Var One = Self [Operator] Var Two
Var One [Operator]= Var Two
036 – Comparison Operators#
[ == ] Equal
[ != ] Not Equal
[ > ] Greater Than
[ < ] Less Than
[ >= ] Greater Than Or Equal
[ <= ] Less Than Or Equal
print(100 == 100)
print(100 == 200)
print(100 == 100.00)
True
False
True
print(100 != 100)
print(100 != 200)
print(100 != 100.00)
False
True
False
print(100 > 100)
print(100 > 200)
print(100 > 100.00)
print(100 > 40)
False
False
False
True
print(100 < 100)
print(100 < 200)
print(100 < 100.00)
print(100 < 40)
False
True
False
False
print(100 >= 100)
print(100 >= 200)
print(100 >= 100.00)
print(100 >= 40)
True
False
True
True
print(100 <= 100)
print(100 <= 200)
print(100 <= 100.00)
print(100 <= 40)
True
True
True
False
037 – Type Conversion#
a = 10
print(type(a))
print(type(str(a)))
<class 'int'>
<class 'str'>
c = "Osama"
d = [1, 2, 3, 4, 5]
e = {"A", "B", "C"}
f = {"A": 1, "B": 2}
print(tuple(c))
print(tuple(d))
print(tuple(e))
print(tuple(f))
('O', 's', 'a', 'm', 'a')
(1, 2, 3, 4, 5)
('B', 'A', 'C')
('A', 'B')
c = "Osama"
d = (1, 2, 3, 4, 5)
e = {"A", "B", "C"}
f = {"A": 1, "B": 2}
print(list(c))
print(list(d))
print(list(e))
print(list(f))
['O', 's', 'a', 'm', 'a']
[1, 2, 3, 4, 5]
['B', 'A', 'C']
['A', 'B']
c = "Osama"
d = (1, 2, 3, 4, 5)
e = ["A", "B", "C"]
f = {"A": 1, "B": 2}
print(set(c))
print(set(d))
print(set(e))
print(set(f))
{'m', 's', 'O', 'a'}
{1, 2, 3, 4, 5}
{'B', 'A', 'C'}
{'B', 'A'}
d = (("A", 1), ("B", 2), ("C", 3))
e = [["One", 1], ["Two", 2], ["Three", 3]]
print(dict(d))
print(dict(e))
{'A': 1, 'B': 2, 'C': 3}
{'One': 1, 'Two': 2, 'Three': 3}
038 – User Input#
fName = input('What\'s Is Your First Name?')
mName = input('What\'s Is Your Middle Name?')
lName = input('What\'s Is Your Last Name?')
fName = fName.strip().capitalize()
mName = mName.strip().capitalize()
lName = lName.strip().capitalize()
print(f"Hello {fName} {mName:.1s} {lName} Happy To See You.")
Hello Ahmed H Darwish Happy To See You.
039 – Practical – Email Slice#
email = "Osama@elzero.org"
print(email[:email.index("@")])
Osama
theName = input('What\'s Your Name ?').strip().capitalize()
theEmail = input('What\'s Your Email ?').strip()
theUsername = theEmail[:theEmail.index("@")]
theWebsite = theEmail[theEmail.index("@") + 1:]
print(f"Hello {theName} Your Email Is {theEmail}")
Hello Ahmed Your Email Is ahmed@mail.com
print(f"Your Username Is {theUsername} \nYour Website Is {theWebsite}")
Your Username Is ahmed
Your Website Is mail.com
040 – Practical – Your Age In Full Details#
age = int(input('What\'s Your Age ? ').strip())
months = age * 12
weeks = months * 4
days = age * 365
hours = days * 24
minutes = hours * 60
seconds = minutes * 60
print('You Lived For:')
print(f"{months} Months.")
print(f"{weeks:,} Weeks.")
print(f"{days:,} Days.")
print(f"{hours:,} Hours.")
print(f"{minutes:,} Minutes.")
print(f"{seconds:,} Seconds.")
You Lived For:
252 Months.
1,008 Weeks.
7,665 Days.
183,960 Hours.
11,037,600 Minutes.
662,256,000 Seconds.
Week 6#
041 - Control Flow If, Elif, Else#
uName = "Osama"
uCountry = "Kuwait"
cName = "Python Course"
cPrice = 100
if uCountry == "Egypt":
print(f"Hello {uName} Because You Are From {uCountry}")
print(f"The Course \"{cName}\" Price Is: ${cPrice - 80}")
elif uCountry == "KSA":
print(f"Hello {uName} Because You Are From {uCountry}")
print(f"The Course \"{cName}\" Price Is: ${cPrice - 60}")
elif uCountry == "Kuwait":
print(f"Hello {uName} Because You Are From {uCountry}")
print(f"The Course \"{cName}\" Price Is: ${cPrice - 50}")
else:
print(f"Hello {uName} Because You Are From {uCountry}")
print(f"The Course \"{cName}\" Price Is: ${cPrice - 30}")
Hello Osama Because You Are From Kuwait
The Course "Python Course" Price Is: $50
042 - Control Flow Nested If and Trainings#
uName = "Osama"
isStudent = "Yes"
uCountry = "Egypt"
cName = "Python Course"
cPrice = 100
if uCountry == "Egypt" or uCountry == "KSA" or uCountry == "Qatar":
if isStudent == "Yes":
print(f"Hi {uName} Because U R From {uCountry} And Student")
print(f"The Course \"{cName}\" Price Is: ${cPrice - 90}")
else:
print(f"Hi {uName} Because U R From {uCountry}")
print(f"The Course \"{cName}\" Price Is: ${cPrice - 80}")
elif uCountry == "Kuwait" or uCountry == "Bahrain":
print(f"Hi {uName} Because U R From {uCountry}")
print(f"The Course \"{cName}\" Price Is: ${cPrice - 50}")
else:
print(f"Hi {uName} Because U R From {uCountry}")
print(f"The Course \"{cName}\" Price Is: ${cPrice - 30}")
Hi Osama Because U R From Egypt And Student
The Course "Python Course" Price Is: $10
043 – Control Flow – Ternary Conditional Operator#
country = "A"
if country == "Egypt":
print(f"The Weather in {country} Is 15")
elif country == "KSA":
print(f"The Weather in {country} Is 30")
else:
print("Country is Not in The List")
Country is Not in The List
movieRate = 18
age = 16
if age < movieRate:
print("Movie S Not Good 4U") # Condition If True
else:
print("Movie S Good 4U And Happy Watching") # Condition If False
Movie S Not Good 4U
print("Movie S Not Good 4U" if age <
movieRate else "Movie S Good 4U And Happy Watching")
Movie S Not Good 4U
Condition If True | If Condition | Else | Condition If False
044 – Calculate Age Advanced Version And Training#
print("#" * 80)
print(" You Can Write The First Letter Or Full Name of The Time Unit ".center(80, '#'))
print("#" * 80)
################################################################################
######### You Can Write The First Letter Or Full Name of The Time Unit #########
################################################################################
# Collect Age Data
age = input("Please Write Your Age").strip()
# Collect Time Unit Data
unit = input("Please Choose Time Unit: Months, Weeks, Days ").strip().lower()
# Get Time Units
months = int(age) * 12
weeks = months * 4
days = int(age) * 365
if unit == 'months' or unit == 'm':
print("You Choosed The Unit Months")
print(f"You Lived For {months:,} Months.")
elif unit == 'weeks' or unit == 'w':
print("You Choosed The Unit Weeks")
print(f"You Lived For {weeks:,} Weeks.")
elif unit == 'days' or unit == 'd':
print("You Choosed The Unit Days")
print(f"You Lived For {days:,} Days.")
You Choosed The Unit Days
You Lived For 7,665 Days.
045 – Membership Operators#
# String
name = "Osama"
print("s" in name)
print("a" in name)
print("A" in name)
True
True
False
# List
friends = ["Ahmed", "Sayed", "Mahmoud"]
print("Osama" in friends)
print("Sayed" in friends)
print("Mahmoud" not in friends)
False
True
False
# Using In and Not In With Condition
countriesOne = ["Egypt", "KSA", "Kuwait", "Bahrain", "Syria"]
countriesOneDiscount = 80
countriesTwo = ["Italy", "USA"]
countriesTwoDiscount = 50
myCountry = "Italy"
if myCountry in countriesOne:
print(f"Hello You Have A Discount Equal To ${countriesOneDiscount}")
elif myCountry in countriesTwo:
print(f"Hello You Have A Discount Equal To ${countriesTwoDiscount}")
else:
print("You Have No Discount")
Hello You Have A Discount Equal To $50
046 - Practical Membership Control#
# List Contains Admins
admins = ["Ahmed", "Osama", "Sameh", "Manal", "Rahma", "Mahmoud", "Enas"]
# Login
name = input("Please Type Your Name ").strip().capitalize()
# If Name is In Admin
if name in admins:
print(f"Hello {name}, Welcome Back.")
option = input("Delete Or Update Your Name ?").strip().capitalize()
# Update Option
if option == 'Update' or option == 'U':
theNewName = input("Your New Name Please ").strip().capitalize()
admins[admins.index(name)] = theNewName
print("Name Updated.")
print(admins)
# Delete Option
elif option == 'Delete' or option == 'D':
admins.remove(name)
print("Name Deleted")
print(admins)
# Wrong Option
else:
print("Wrong Option Choosed")
# If Name is not In Admin
else:
status = input("Not Admin, Add You Y, N ? ").strip().capitalize()
if status == "Yes" or status == "Y":
print("You Have Been Added")
admins.append(name)
print(admins)
else:
print("You Are Not Added.")
Hello Ahmed, Welcome Back.
Name Deleted
['Osama', 'Sameh', 'Manal', 'Rahma', 'Mahmoud', 'Enas']
Week 7#
047 - Loop – While and Else#
while condition_is_true
Code Will Run Until Condition Become False```
a = 10
while a < 15:
print(a)
a += 1 # a = a + 1
else:
print("Loop is Done") # True Become False
while False:
print("Will Not Print")
10
11
12
13
14
Loop is Done
a = 10
while a < 15:
print(a)
a += 1 # a = a + 1
print("Loop is Done") # True Become False
while False:
print("Will Not Print")
10
11
12
13
14
Loop is Done
a = 15
while a < 15:
print(a)
a += 1 # a = a + 1
print("Loop is Done") # True Become False
while False:
print("Will Not Print")
Loop is Done
048 – Loop – While Trainings#
myF = ["Os", "Ah", "Ga", "Al", "Ra", "Sa", "Ta", "Ma", "Mo", "Wa"]
print(myF)
['Os', 'Ah', 'Ga', 'Al', 'Ra', 'Sa', 'Ta', 'Ma', 'Mo', 'Wa']
print(myF[0])
print(myF[1])
print(myF[2])
print(myF[9])
Os
Ah
Ga
Wa
print(len(myF))
10
a = 0
while a < len(myF): # a < 10
print(myF[a])
a += 1 # a = a + 1
else:
print("All Friends Printed To Screen.")
Os
Ah
Ga
Al
Ra
Sa
Ta
Ma
Mo
Wa
All Friends Printed To Screen.
a = 0
while a < len(myF): # a < 10
# a+1 to make zfill start with 001
print(f"#{str(a + 1).zfill(2)} {myF[a]}")
a += 1 # a = a + 1
else:
print("All Friends Printed To Screen.")
#01 Os
#02 Ah
#03 Ga
#04 Al
#05 Ra
#06 Sa
#07 Ta
#08 Ma
#09 Mo
#10 Wa
All Friends Printed To Screen.
049 – Loop – While Trainings Bookmark Manager#
# Empty List To Fill Later
myFavouriteWebs = []
# Maximum Allowed Websites
maximumWebs = 2
while maximumWebs > 0:
# Input The New Website
web = input("Website Name Without https:// ")
# Add The New Website To The List
myFavouriteWebs.append(f"https://{web.strip().lower()}")
# Decrease One Number From Allowed Websites
maximumWebs -= 1 # maximumWebs = maximumWebs - 1
# Print The Add Message
print(f"Website Added, {maximumWebs} Places Left")
# Print The List
print(myFavouriteWebs)
else:
print("Bookmark Is Full, You Cant Add More")
Website Added, 1 Places Left
['https://elzero.org']
Website Added, 0 Places Left
['https://elzero.org', 'https://']
Bookmark Is Full, You Cant Add More
# Check If List Is Not Empty
if len(myFavouriteWebs) > 0:
# Sort The List
myFavouriteWebs.sort()
index = 0
print("Printing The List Of Websites in Your Bookmark")
while index < len(myFavouriteWebs):
print(myFavouriteWebs[index])
index += 1 # index = index + 1
Printing The List Of Websites in Your Bookmark
https://
https://elzero.org
050 – Loop – While Trainings Password Guess#
tries = 2
mainPassword = "123"
inputPassword = input("Write Your Password: ")
while inputPassword != mainPassword: # True
tries -= 1 # tries = tries - 1
print(f"Wrong Password, { 'Last' if tries == 0 else tries } Chance Left")
inputPassword = input("Write Your Password: ")
if tries == 0:
print("All Tries Is Finished.")
break # exits the loop
print("Will Not Print") # will not be printed
else:
print("Correct Password")
Wrong Password, 1 Chance Left
Wrong Password, Last Chance Left
All Tries Is Finished.
051 – Loop – For And Else#
for item in iterable_object :
Do Something With Item
item Is A Vairable You Create and Call Whenever You Want
item refer to the current position and will run and visit all items to the end
iterable_object => Sequence [ list, tuples, set, dict, string of charcaters, etc … ]
myNumbers = [1, 2, 3, 4, 5]
print(myNumbers)
[1, 2, 3, 4, 5]
for number in myNumbers:
print(number)
1
2
3
4
5
# check if the number is even.
for number in myNumbers:
if number % 2 == 0: # Even
print(f"The Number {number} Is Even.")
else:
print(f"The Number {number} Is Odd.")
else:
print("The Loop Is Finished")
The Number 1 Is Odd.
The Number 2 Is Even.
The Number 3 Is Odd.
The Number 4 Is Even.
The Number 5 Is Odd.
The Loop Is Finished
myName = "Osama"
for letter in myName:
print(letter)
O
s
a
m
a
myName = "Osama"
for letter in myName:
print(f" [ {letter.upper()} ] ")
[ O ]
[ S ]
[ A ]
[ M ]
[ A ]
052 – Loop – For Trainings#
myRange = range(0, 6)
for number in myRange:
print(number)
0
1
2
3
4
5
for number in range(6):
print(number)
0
1
2
3
4
5
mySkills = {
"Html": "90%",
"Css": "60%",
"PHP": "70%",
"JS": "80%",
"Python": "90%",
"MySQL": "60%"
}
print(mySkills['JS'])
print(mySkills.get("Python"))
80%
90%
# looping on dictionary
# will print the keys
for skill in mySkills:
print(skill)
Html
Css
PHP
JS
Python
MySQL
for skill in mySkills:
print(f"My Progress in Lang {skill} Is: {mySkills[skill]}")
My Progress in Lang Html Is: 90%
My Progress in Lang Css Is: 60%
My Progress in Lang PHP Is: 70%
My Progress in Lang JS Is: 80%
My Progress in Lang Python Is: 90%
My Progress in Lang MySQL Is: 60%
053 – Loop – For Nested Loop#
people = ["Osama", "Ahmed", "Sayed", "Ali"]
skills = ['Html', 'Css', 'Js']
for name in people: # Outer Loop
print(f"{name} Skills are: ")
for skill in skills: # Inner Loop
print(f"- {skill}")
Osama Skills are:
- Html
- Css
- Js
Ahmed Skills are:
- Html
- Css
- Js
Sayed Skills are:
- Html
- Css
- Js
Ali Skills are:
- Html
- Css
- Js
people = {
"Osama": {
"Html": "70%",
"Css": "80%",
"Js": "70%"
},
"Ahmed": {
"Html": "90%",
"Css": "80%",
"Js": "90%"
},
"Sayed": {
"Html": "70%",
"Css": "60%",
"Js": "90%"
}
}
print(people["Ahmed"])
print(people["Ahmed"]['Css'])
{'Html': '90%', 'Css': '80%', 'Js': '90%'}
80%
for name in people:
print(f"Skills and Progress For {name} are: ")
for skill in people[name]:
print(f"{skill.upper()} => {people[name][skill]}")
Skills and Progress For Osama are:
HTML => 70%
CSS => 80%
JS => 70%
Skills and Progress For Ahmed are:
HTML => 90%
CSS => 80%
JS => 90%
Skills and Progress For Sayed are:
HTML => 70%
CSS => 60%
JS => 90%
054 – Break, Continue, Pass#
myNumbers = [1, 2, 3, 4, 5]
for number in myNumbers:
if number == 2:
continue # skips this number
print(number)
1
3
4
5
for number in myNumbers:
if number == 2:
break # will stop the loop
print(number)
1
for number in myNumbers:
if number == 2:
pass # will add a feature later, go on for now
print(number)
1
2
3
4
5
055 – Loop Advanced Dictionary#
mySkills = {
"HTML": "80%",
"CSS": "90%",
"JS": "70%",
"PHP": "80%"
}
print(mySkills.items())
dict_items([('HTML', '80%'), ('CSS', '90%'), ('JS', '70%'), ('PHP', '80%')])
for skill in mySkills:
print(f"{skill} => {mySkills[skill]}")
HTML => 80%
CSS => 90%
JS => 70%
PHP => 80%
for skill_key, skill_progress in mySkills.items():
print(f"{skill_key} => {skill_progress}")
HTML => 80%
CSS => 90%
JS => 70%
PHP => 80%
myUltimateSkills = {
"HTML": {
"Main": "80%",
"Pugjs": "80%"
},
"CSS": {
"Main": "90%",
"Sass": "70%"
}
}
for main_key, main_value in myUltimateSkills.items():
print(f"{main_key} Progress Is: ")
for child_key, child_value in main_value.items():
print(f"- {child_key} => {child_value}")
HTML Progress Is:
- Main => 80%
- Pugjs => 80%
CSS Progress Is:
- Main => 90%
- Sass => 70%
Week 8#
056 – Function and Return#
[1] A Function is A Reusable Block Of Code Do A Task
[2] A Function Run When You Call It
[3] A Function Accept Element To Deal With Called [Parameters]
[4] A Function Can Do The Task Without Returning Data
[5] A Function Can Return Data After Job is Finished
[6] A Function Create To Prevent DRY
[7] A Function Accept Elements When You Call It Called [Arguments]
[8] There’s A Built-In Functions and User Defined Functions
[9] A Function Is For All Team and All Apps
def function_name():
return "Hello Python From Inside Function"
dataFromFunction = function_name()
print(dataFromFunction)
Hello Python From Inside Function
def function_name():
print("Hello Python From Inside Function")
function_name()
Hello Python From Inside Function
057 – Function Parameters And Arguments#
a, b, c = "Osama", "Ahmed", "Sayed"
print(f"Hello {a}")
print(f"Hello {b}")
print(f"Hello {c}")
Hello Osama
Hello Ahmed
Hello Sayed
# def => Function Keyword [Define]
# say_hello() => Function Name
# name => Parameter
# print(f"Hello {name}") => Task
# say_hello("Ahmed") => Ahmed is The Argument
def say_hello(name):
print(f"Hello {name}")
say_hello("ahmed")
Hello ahmed
def say_hello(n):
print(f"Hello {n}")
say_hello("ahmed")
Hello ahmed
a, b, c = "Osama", "Ahmed", "Sayed"
say_hello(a)
say_hello(b)
say_hello(c)
Hello Osama
Hello Ahmed
Hello Sayed
def addition(n1, n2):
print(n1 + n2)
addition(20, 50) # must be two arguements
70
def addition(n1, n2):
if type(n1) != int or type(n2) != int:
print("Only integers allowed")
else:
print(n1 + n2)
addition(20, "ahmed")
Only integers allowed
def full_name(first, middle, last):
print(
f"Hello {first.strip().capitalize()} {middle.upper():.1s} {last.capitalize()} ")
full_name(" osama ", 'mohamed', "elsayed")
Hello Osama M Elsayed
058 – Function Packing, Unpacking Arguments#
print(1, 2, 3, 4)
1 2 3 4
myList = [1, 2, 3, 4]
print(myList) # list
print(*myList) # unpack the list
[1, 2, 3, 4]
1 2 3 4
def say_hello(n1, n2, n3, n4):
peoples = [n1, n2, n3, n4]
for name in peoples:
print(f"Hello {name}")
say_hello("Osama", "Ahmed", "Sayed", "Mahmoud") # must be 4 names
Hello Osama
Hello Ahmed
Hello Sayed
Hello Mahmoud
def say_hello(*peoples):
for name in peoples:
print(f"Hello {name}")
say_hello("ahmed", "darwish") # any number of people
Hello ahmed
Hello darwish
def show_details(skill1, skill2, skill3):
print(f"hello osama, your skills are: ")
print(f"{skill1}")
print(f"{skill2}")
print(f"{skill3}")
show_details("html", "css", "js") # must be three
hello osama, your skills are:
html
css
js
def show_details(*skills):
print(f"hello osama, your skills are: ")
for skill in skills:
print(skill)
show_details("html", "css", "js", "python")
hello osama, your skills are:
html
css
js
python
def show_details(name, *skills):
print(f"hello {name}, your skills are: ")
for skill in skills:
print(skill, end=" ")
show_details("ahmed", "html", "css", "js", "python")
show_details("osama", "js")
hello ahmed, your skills are:
html css js python hello osama, your skills are:
js
059 - Function Default Parameters#
def say_hello(name, age, country):
print(f"Hello {name} Your Age is {age} and Your Country Is {country}")
say_hello("Osama", 36, "Egypt")
say_hello("Mahmoud", 28, "KSA") # must enter 3 arguements
Hello Osama Your Age is 36 and Your Country Is Egypt
Hello Mahmoud Your Age is 28 and Your Country Is KSA
def say_hello(name="Unknown", age="Unknown", country="Unknown"):
print(f"Hello {name} Your Age is {age} and Your Country Is {country}")
say_hello("Osama", 36, "Egypt")
say_hello("Mahmoud", 28, "KSA")
say_hello("Sameh", 38)
say_hello("Ramy")
say_hello() # prints unknown if not provided
# if you provide only one default,it must be the last parameter
# def say_hello(name="Unknown", age, country): error
Hello Osama Your Age is 36 and Your Country Is Egypt
Hello Mahmoud Your Age is 28 and Your Country Is KSA
Hello Sameh Your Age is 38 and Your Country Is Unknown
Hello Ramy Your Age is Unknown and Your Country Is Unknown
Hello Unknown Your Age is Unknown and Your Country Is Unknown
060 – Function Packing, Unpacking Keyword Arguments#
def show_skills(*skills):
for skill in skills:
print(f"{skill}")
show_skills("Html", "CSS", "JS")
Html
CSS
JS
def show_skills(*skills):
print(type(skills)) # tuple
for skill in skills:
print(f"{skill}")
show_skills("Html", "CSS", "JS")
<class 'tuple'>
Html
CSS
JS
def show_skills(**skills):
print(type(skills)) # dict
for skill, value in skills.items():
print(f"{skill} => {value}")
mySkills = {
'Html': "80%",
'Css': "70%",
'Js': "50%",
'Python': "80%",
"Go": "40%"
}
show_skills(**mySkills)
print("---------------")
show_skills(Html="80%", CSS="70%", JS="50%")
<class 'dict'>
Html => 80%
Css => 70%
Js => 50%
Python => 80%
Go => 40%
---------------
<class 'dict'>
Html => 80%
CSS => 70%
JS => 50%
061 – Function Packing, Unpacking Arguments Trainings#
def show_skills(name, *skills):
print(f"Hello {name}\nskills without progress are: ")
for skill in skills:
print(f"- {skill}")
show_skills("osama", "html", "css", "js")
Hello osama
skills without progress are:
- html
- css
- js
def show_skills(name, *skills, **skillsWithProgres):
print(f"Hello {name} \nSkills Without Progress are: ")
for skill in skills:
print(f"- {skill}")
print("Skills With Progress are: ")
for skill_key, skill_value in skillsWithProgres.items():
print(f"- {skill_key} => {skill_value}")
show_skills("osama")
print("-----------")
show_skills("Osama", "html", "css", "js", python="50%")
Hello osama
Skills Without Progress are:
Skills With Progress are:
-----------
Hello Osama
Skills Without Progress are:
- html
- css
- js
Skills With Progress are:
- python => 50%
myTuple = ("Html", "CSS", "JS")
mySkills = {
'Go': "80%",
'Python': "50%",
'MySQL': "80%"
}
show_skills("Osama", *myTuple, **mySkills)
Hello Osama
Skills Without Progress are:
- Html
- CSS
- JS
Skills With Progress are:
- Go => 80%
- Python => 50%
- MySQL => 80%
062 – Function Scope#
x = 1
print(f"varible in global scope: {x}")
varible in global scope: 1
x = 1
def one():
x = 2
print(f"variable in function scope: {x}")
print(f"varible in global scope: {x}")
one()
varible in global scope: 1
variable in function scope: 2
x = 1
def one():
x = 2
print(f"variable in function one scope: {x}")
def two():
x = 4
print(f"variable in function two scope: {x}")
print(f"varible in global scope: {x}")
one()
two()
varible in global scope: 1
variable in function one scope: 2
variable in function two scope: 4
x = 1
def one():
x = 2
print(f"variable in function one scope: {x}")
def two():
print(f"variable in function two scope: {x}") # from global
print(f"varible in global scope: {x}")
one()
two()
varible in global scope: 1
variable in function one scope: 2
variable in function two scope: 1
x = 1
def one():
global x
x = 2
print(f"variable in function one scope: {x}")
def two():
x = 4
print(f"variable in function two scope: {x}")
print(f"varible in global scope: {x}")
one()
two()
print(f"varible in global scope after function one: {x}")
varible in global scope: 1
variable in function one scope: 2
variable in function two scope: 4
varible in global scope after function one: 2
def one():
global t
t = 10
print(f"variable in function one scope: {t}")
def two():
print(f"variable in function two scope: {t}")
# print(f"varible in global scope: {x}") error
one()
two()
print(f"varible in global scope after function one: {t}")
variable in function one scope: 10
variable in function two scope: 10
varible in global scope after function one: 10
063 – Function Recursion#
x = "wooorrldd"
print(x[1:])
ooorrldd
def cleanWord(word):
if len(word) == 1:
return word
if word[0] == word[1]:
return cleanWord(word[1:])
return word
print(cleanWord("wwwoorrlldd"))
woorrlldd
def cleanWord(word):
if len(word) == 1:
return word
if word[0] == word[1]:
return cleanWord(word[1:])
return word[0] + cleanWord(word[1:])
# Stash [ World ]
print(cleanWord("wwwoorrlldd"))
world
def cleanWord(word):
if len(word) == 1:
return word
print(f"print start function {word}")
if word[0] == word[1]:
print(f"print before condition {word}")
return cleanWord(word[1:])
print(f"before return {word}")
print("-----------------------")
return word[0] + cleanWord(word[1:])
# Stash [ World ]
print(cleanWord("wwwoorrlldd"))
print start function wwwoorrlldd
print before condition wwwoorrlldd
print start function wwoorrlldd
print before condition wwoorrlldd
print start function woorrlldd
before return woorrlldd
-----------------------
print start function oorrlldd
print before condition oorrlldd
print start function orrlldd
before return orrlldd
-----------------------
print start function rrlldd
print before condition rrlldd
print start function rlldd
before return rlldd
-----------------------
print start function lldd
print before condition lldd
print start function ldd
before return ldd
-----------------------
print start function dd
print before condition dd
world
064 – Function Lambda#
[1] It Has No Name
[2] You Can Call It Inline Without Defining It
[3] You Can Use It In Return Data From Another Function
[4] Lambda Used For Simple Functions and Def Handle The Large Tasks
[5] Lambda is One Single Expression not Block Of Code
[6] Lambda Type is Function
def say_hello(name, age): return f"Hello {name} your Age Is: {age}"
print(say_hello("Ahmed", 36))
def hello(name, age): return f"Hello {name} your Age Is: {age}"
print(hello("Ahmed", 36))
Hello Ahmed your Age Is: 36
Hello Ahmed your Age Is: 36
print(say_hello.__name__)
print(hello.__name__)
say_hello
hello
print(type(say_hello))
print(type(hello))
<class 'function'>
<class 'function'>
Week 9#
065 - Files Handling – Part 1 Intro#
“a” Append Open File For Appending Values, Create File If Not Exists
“r” Read [Default Value] Open File For Read and Give Error If File is Not Exists
“w” Write Open File For Writing, Create File If Not Exists
“x” Create Create File, Give Error If File Exists
file = open(“D:\Python\Files\osama.txt”)
import os # operating system
print(os.getcwd()) # main current working directory
f:\github\python\Mastering-Python
print(os.path.abspath(file)) # absolute path of file
directory for the opened files
print(os.path.dirname(os.path.abspath(file)))
change current working directory
os.chdir(os.path.dirname(os.path.abspath(file))) print(os.getcwd())
r
will make sure \n
is not treated as new line
file = open(r”D:\Python\Files\nfiles\osama.txt”)
066 – Files Handling Part 2 – Read Files#
myfile = open(r"D:\Python\Files\osama.txt", "r")
print(myfile) # file data object
print(myfile.name)
print(myfile.mode)
print(myfile.encoding)
print(myfile.read())
print(myfile.read(5))
print(myfile.readline(5))
print(myfile.readline())
print(myfile.readlines(50))
print(myfile.readlines())
print(type(myfile.readlines()))
for line in myfile:
print(line)
if line.startswith("07"):
break
myfile.close()
067 – Files Handling Part 3 – Write And Append In Files#
myfile = open(r"D:\Python\Files\osama.txt", "w")
myfile.write("hello\n")
myfile.write("third line")
myfile.write("elzero web school\n" * 1000)
mylist = ["osama\n", "ahmed\n", "sayed\n"]
myfiles.writelines(mylist)
myfile = open(r"D:\Python\Files\osama.txt", "a")
myfile.write("osama")
068 – Files Handling Part 4 – Important Informations#
import os
myFile = open("D:\Python\Files\osama.txt", "a")
myFile.truncate(5)
myFile = open("D:\Python\Files\osama.txt", "a")
print(myFile.tell())
myFile = open("D:\Python\Files\osama.txt", "r")
myFile.seek(11)
print(myFile.read())
os.remove("D:\Python\Files\osama.txt")
Week 10#
069 – Built In Functions Part 1#
x = [1, 2, 3, 4, []]
if all(x):
print("All Elements Is True")
else:
print("at least one is false")
at least one is false
x = [1, 2, 3, 4, []]
if any(x):
print("at least one is true")
else:
print("Theres No Any True Elements")
at least one is true
x = [False, 0, {}, (), []]
if any(x):
print("at least one is true")
else:
print("Theres No Any True Elements")
Theres No Any True Elements
print(bin(100))
0b1100100
a = 1
b = 2
print(id(a))
print(id(b))
140715333358376
140715333358408
070 - Built In Functions Part 2#
# sum(iterable, start)
a = [1, 10, 19, 40]
print(sum(a))
print(sum(a, 5))
70
75
# round(number, numofdigits)
print(round(150.499))
print(round(150.555, 2))
print(round(150.554, 2))
150
150.56
150.55
# range(start, end, step)
print(list(range(0)))
print(list(range(10)))
print(list(range(0, 10, 2)))
[]
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
[0, 2, 4, 6, 8]
# print()
print("Hello Osama")
print("Hello", "Osama")
Hello Osama
Hello Osama
print("Hello @ Osama @ How @ Are @ You")
print("Hello", "Osama", "How", "Are", "You", sep=" @ ")
Hello @ Osama @ How @ Are @ You
Hello @ Osama @ How @ Are @ You
print("First Line", end="\n")
print("Second Line", end=" ")
print("Third Line")
First Line
Second Line Third Line
071 - Built In Functions Part 3#
# abs()
print(abs(100))
print(abs(-100))
print(abs(10.19))
print(abs(-10.19))
100
100
10.19
10.19
# pow(base, exp, mod)
print(pow(2, 5))
print(pow(2, 5, 10))
32
2
# min(item, item , item, or iterator)
myNumbers = (1, 20, -50, -100, 100)
print(min(1, 10, -50, 20, 30))
print(min("X", "Z", "Osama"))
print(min(myNumbers))
-50
Osama
-100
# max(item, item , item, or iterator)
myNumbers = (1, 20, -50, -100, 100)
print(max(1, 10, -50, 20, 30))
print(max("X", "Z", "Osama"))
print(max(myNumbers))
30
Z
100
# slice(start, end, step)
a = ["A", "B", "C", "D", "E", "F"]
print(a[:5])
print(a[slice(5)])
print(a[slice(2, 5)])
['A', 'B', 'C', 'D', 'E']
['A', 'B', 'C', 'D', 'E']
['C', 'D', 'E']
072 – Built In Functions Part 4 – Map#
[1] Map Take A Function + Iterator
[2] Map Called Map Because It Map The Function On Every Element
[3] The Function Can Be Pre-Defined Function or Lambda Function
# map with predefined function
def formatText(text):
return f"- {text.strip().capitalize()}-"
myTexts = ["osama", "ahmed"]
myFormatedData = map(formatText, myTexts)
print(myFormatedData)
<map object at 0x000002B95FCDA3E0>
for name in map(formatText, myTexts):
print(name)
- Osama-
- Ahmed-
for name in list(map(formatText, myTexts)):
print(name)
- Osama-
- Ahmed-
# map with lambda
myTexts = ["osama", "ahmed"]
for name in list(map(lambda text: f"- {text.strip().capitalize()}-", myTexts)):
print(name)
- Osama-
- Ahmed-
# map with lambda
myTexts = ["osama", "ahmed"]
for name in list(map((lambda text: f"- {text.strip().capitalize()}-"), myTexts)):
print(name)
- Osama-
- Ahmed-
073 - Built In Functions Part 5 – Filter#
[1] Filter Take A Function + Iterator
[2] Filter Run A Function On Every Element
[3] The Function Can Be Pre-Defined Function or Lambda Function
[4] Filter Out All Elements For Which The Function Return True
[5] The Function Need To Return Boolean Value
def checkNumber(num):
if num > 10:
return num
myNumbers = [1, 19, 10, 20, 100, 5]
myResult = filter(checkNumber, myNumbers)
for number in myResult:
print(number)
19
20
100
def checkNumber(num):
if num == 0:
return num # wont work cuz 0 is falsy
myNumbers = [0, 0, 1, 19, 10, 20, 100, 5, 0, 0]
myResult = filter(checkNumber, myNumbers)
for number in myResult:
print(number)
def checkNumber(num):
if num == 0:
return True
myNumbers = [0, 0, 1, 19, 10, 20, 100, 5, 0, 0]
myResult = filter(checkNumber, myNumbers)
for number in myResult:
print(number)
0
0
0
0
def checkNumber(num):
return num > 10
myNumbers = [1, 19, 10, 20, 100, 5]
myResult = filter(checkNumber, myNumbers)
for number in myResult:
print(number)
19
20
100
def checkName(name):
return name.startswith("O")
myTexts = ["Osama", "Omer", "Omar", "Ahmed", "Sayed", "Othman"]
myReturnedData = filter(checkName, myTexts)
for person in myReturnedData:
print(person)
Osama
Omer
Omar
Othman
myNames = ["Osama", "Omer", "Omar", "Ahmed", "Sayed", "Othman", "Ameer"]
for p in filter(lambda name: name.startswith("A"), myNames):
print(p)
Ahmed
Ameer
074 – Built In Functions Part 6 – Reduce#
[1] Reduce Take A Function + Iterator
[2] Reduce Run A Function On FIrst and Second Element And Give Result
[3] Then Run Function On Result And Third Element
[4] Then Run Function On Rsult And Fourth Element And So On
[5] Till One ELement is Left And This is The Result of The Reduce
[6] The Function Can Be Pre-Defined Function or Lambda Function
from functools import reduce
def sumAll(num1, num2):
return num1 + num2
numbers = [5, 10, 15, 20, 30]
result = reduce(sumAll, numbers)
print(result)
print(((((5+10)+15)+20)+30))
80
80
result = reduce(lambda num1, num2: num1 + num2, numbers)
print(result)
80
075 – Built In Functions Part 7#
# enumerate(iterable, start=0)
mySkills = ["Html", "Css", "Js", "PHP"]
for skill in mySkills:
print(skill)
Html
Css
Js
PHP
mySkillsWithCounter = enumerate(mySkills, 00)
print(type(mySkillsWithCounter))
for skill in mySkillsWithCounter:
print(skill)
<class 'enumerate'>
(0, 'Html')
(1, 'Css')
(2, 'Js')
(3, 'PHP')
mySkillsWithCounter = enumerate(mySkills, 00)
for counter, skill in mySkillsWithCounter:
print(f"{counter} - {skill}")
0 - Html
1 - Css
2 - Js
3 - PHP
print(help(print))
Help on built-in function print in module builtins:
print(*args, sep=' ', end='\n', file=None, flush=False)
Prints the values to a stream, or to sys.stdout by default.
sep
string inserted between values, default a space.
end
string appended after the last value, default a newline.
file
a file-like object (stream); defaults to the current sys.stdout.
flush
whether to forcibly flush the stream.
None
# reversed(iterable)
myString = "Elzero"
print(reversed(myString))
<reversed object at 0x000002B95FCDA560>
for letter in reversed(myString):
print(letter, end="")
orezlE
for s in reversed(mySkills):
print(s)
PHP
Js
Css
Html
Week 11#
076 – Modules Part 1 – Intro And Built In Modules#
[1] Module is A File Contain A Set Of Functions
[2] You Can Import Module in Your App To Help You
[3] You Can Import Multiple Modules
[4] You Can Create Your Own Modules
[5] Modules Saves Your Time
import random
print(random)
<module 'random' from 'f:\\miniconda3\\envs\\py311\\Lib\\random.py'>
print(f"random float number {random.random()}")
random float number 0.8022768368311108
# show all functions inside module
import random
print(dir(random))
['BPF', 'LOG4', 'NV_MAGICCONST', 'RECIP_BPF', 'Random', 'SG_MAGICCONST', 'SystemRandom', 'TWOPI', '_ONE', '_Sequence', '_Set', '__all__', '__builtins__', '__cached__', '__doc__', '__file__', '__loader__', '__name__', '__package__', '__spec__', '_accumulate', '_acos', '_bisect', '_ceil', '_cos', '_e', '_exp', '_floor', '_index', '_inst', '_isfinite', '_log', '_os', '_pi', '_random', '_repeat', '_sha512', '_sin', '_sqrt', '_test', '_test_generator', '_urandom', '_warn', 'betavariate', 'choice', 'choices', 'expovariate', 'gammavariate', 'gauss', 'getrandbits', 'getstate', 'lognormvariate', 'normalvariate', 'paretovariate', 'randbytes', 'randint', 'random', 'randrange', 'sample', 'seed', 'setstate', 'shuffle', 'triangular', 'uniform', 'vonmisesvariate', 'weibullvariate']
# import functions from module
from random import randint, random
print(f"random integer: {randint(100,900)}")
print(f"float number: {random()}")
random integer: 484
float number: 0.9107908848337325
to import all functions from a module
from random import *
077 - Modules – Part 2 – Create Your Module#
first, create your functions in a file (ex: elzero) then:
import sys
print(sys.path)
['f:\\github\\python\\Mastering-Python', 'f:\\miniconda3\\envs\\py311\\python311.zip', 'f:\\miniconda3\\envs\\py311\\DLLs', 'f:\\miniconda3\\envs\\py311\\Lib', 'f:\\miniconda3\\envs\\py311', '', 'f:\\miniconda3\\envs\\py311\\Lib\\site-packages', 'f:\\miniconda3\\envs\\py311\\Lib\\site-packages\\win32', 'f:\\miniconda3\\envs\\py311\\Lib\\site-packages\\win32\\lib', 'f:\\miniconda3\\envs\\py311\\Lib\\site-packages\\Pythonwin']
to add other path (games):
sys.path.append(r”D:\Games”)
import elzero
print(dir(elzero))
elzero.sayHello("Ahmed")
elzero.sayHello("Osama")
elzero.sayHowAreYou("Ahmed")
elzero.sayHowAreYou("Osama")
ALias
import elzero as ee
ee.sayHello("Ahmed")
ee.sayHello("Osama")
ee.sayHowAreYou("Ahmed")
ee.sayHowAreYou("Osama")
from elzero import sayHello
sayHello("Osama")
from elzero import sayHello as ss
ss("Osama")
078 - Modules – Part 3 – Install External Packages#
[1] Module vs Package
[2] External Packages Downloaded From The Internet
[3] You Can Install Packages With Python Package Manager PIP
[4] PIP Install the Package and Its Dependencies
[5] Modules List “https://docs.python.org/3/py-modindex.html”
[6] Packages and Modules Directory “https://pypi.org/”
[7] PIP Manual “https://pip.pypa.io/en/stable/reference/pip_install/”
pip - -version
pip list
pip install termcolor pyfiglet
pip install –user pip –upgrade
import termcolor
import pyfiglet
import textwrap
output = '\n'.join(dir(pyfiglet))
print(textwrap.fill(output, width=80))
COLOR_CODES CharNotPrinted DEFAULT_FONT Figlet FigletBuilder FigletError
FigletFont FigletProduct FigletRenderingEngine FigletString FontError
FontNotFound InvalidColor OptionParser RESET_COLORS SHARED_DIRECTORY __author__
__builtins__ __cached__ __copyright__ __doc__ __file__ __loader__ __name__
__package__ __path__ __spec__ __version__ color_to_ansi figlet_format main os
parse_color pkg_resources print_figlet print_function re shutil sys
unicode_literals unicode_string version zipfile
print(pyfiglet.figlet_format("Ahmed"))
_ _ _
/ \ | |__ _ __ ___ ___ __| |
/ _ \ | '_ \| '_ ` _ \ / _ \/ _` |
/ ___ \| | | | | | | | | __/ (_| |
/_/ \_\_| |_|_| |_| |_|\___|\__,_|
print(termcolor.colored("Ahmed", color="yellow"))
Ahmed
print(termcolor.colored(pyfiglet.figlet_format("Ahmed"), color="yellow"))
_ _ _
/ \ | |__ _ __ ___ ___ __| |
/ _ \ | '_ \| '_ ` _ \ / _ \/ _` |
/ ___ \| | | | | | | | | __/ (_| |
/_/ \_\_| |_|_| |_| |_|\___|\__,_|
079 - Date And Time – Introduction#
import textwrap
import datetime
output = '\n'.join(dir(datetime))
print(textwrap.fill(output, width=80))
MAXYEAR MINYEAR UTC __all__ __builtins__ __cached__ __doc__ __file__ __loader__
__name__ __package__ __spec__ date datetime datetime_CAPI sys time timedelta
timezone tzinfo
import textwrap
output = '\n'.join(dir(datetime.datetime))
print(textwrap.fill(output, width=80))
__add__ __class__ __delattr__ __dir__ __doc__ __eq__ __format__ __ge__
__getattribute__ __getstate__ __gt__ __hash__ __init__ __init_subclass__ __le__
__lt__ __ne__ __new__ __radd__ __reduce__ __reduce_ex__ __repr__ __rsub__
__setattr__ __sizeof__ __str__ __sub__ __subclasshook__ astimezone combine ctime
date day dst fold fromisocalendar fromisoformat fromordinal fromtimestamp hour
isocalendar isoformat isoweekday max microsecond min minute month now replace
resolution second strftime strptime time timestamp timetuple timetz today
toordinal tzinfo tzname utcfromtimestamp utcnow utcoffset utctimetuple weekday
year
# Print The Current Date and Time
print(datetime.datetime.now())
2023-04-22 11:15:04.780122
print(datetime.datetime.now().year)
print(datetime.datetime.now().month)
print(datetime.datetime.now().day)
2023
4
22
# print start and end of date
print(datetime.datetime.min)
print(datetime.datetime.max)
0001-01-01 00:00:00
9999-12-31 23:59:59.999999
# Print The Current Time
print(datetime.datetime.now().time())
print(datetime.datetime.now().time().hour)
print(datetime.datetime.now().time().minute)
print(datetime.datetime.now().time().second)
11:15:07.549813
11
15
7
# Print Start and End Of Time
print(datetime.time.min)
print(datetime.time.max)
00:00:00
23:59:59.999999
# Print Specific Date
print(datetime.datetime(1982, 10, 25))
print(datetime.datetime(1982, 10, 25, 10, 45, 55, 150364))
1982-10-25 00:00:00
1982-10-25 10:45:55.150364
myBirthDay = datetime.datetime(2001, 10, 17)
dateNow = datetime.datetime.now()
print(f"My Birthday is {myBirthDay} And ", end="")
print(f"Date Now Is {dateNow}")
print(f" I Lived For {dateNow - myBirthDay}")
print(f" I Lived For {(dateNow - myBirthDay).days} Days.")
My Birthday is 2001-10-17 00:00:00 And Date Now Is 2023-04-22 11:15:10.227776
I Lived For 7857 days, 11:15:10.227776
I Lived For 7857 Days.
080 - Date And Time – Format Date#
import datetime
myBirthday = datetime.datetime(1982, 10, 25)
print(myBirthday)
print(myBirthday.strftime("%a"))
print(myBirthday.strftime("%A"))
print(myBirthday.strftime("%b"))
print(myBirthday.strftime("%B"))
1982-10-25 00:00:00
Mon
Monday
Oct
October
print(myBirthday.strftime("%d %B %Y"))
print(myBirthday.strftime("%d, %B, %Y"))
print(myBirthday.strftime("%d/%B/%Y"))
print(myBirthday.strftime("%d - %B - %Y"))
print(myBirthday.strftime("%B - %Y"))
25 October 1982
25, October, 1982
25/October/1982
25 - October - 1982
October - 1982
081 - Iterable vs Iterator#
Iterable
[1] Object Contains Data That Can Be Iterated Upon
[2] Examples (String, List, Set, Tuple, Dictionary)
Iterator
[1] Object Used To Iterate Over Iterable Using next() Method Return 1 Element At A Time
[2] You Can Generate Iterator From Iterable When Using iter() Method
[3] For Loop Already Calls iter() Method on The Iterable Behind The Scene
[4] Gives “StopIteration” If Theres No Next Element
myString = "Osama"
for letter in myString:
print(letter, end=" ")
O s a m a
myList = [1, 2, 3, 4, 5]
for number in myList:
print(number, end=" ")
1 2 3 4 5
myNumber = 100.50
for part in myNumber:
print(part) # error
my_name = "ahmed"
my_iterator = iter(my_name)
print(next(my_iterator))
print(next(my_iterator))
print(next(my_iterator))
print(next(my_iterator))
print(next(my_iterator))
a
h
m
e
d
for letter in "Elzero":
print(letter, end=" ")
E l z e r o
for letter in iter("Elzero"):
print(letter, end=" ")
E l z e r o
082 - Generators#
[1] Generator is a Function With “yield” Keyword Instead of “return”
[2] It Support Iteration and Return Generator Iterator By Calling “yield”
[3] Generator Function Can Have one or More “yield”
[4] By Using next() It Resume From Where It Called “yield” Not From Begining
[5] When Called, Its Not Start Automatically, Its Only Give You The Control
def myGenerator():
yield 1
yield 2
yield 3
yield 4
print(myGenerator())
<generator object myGenerator at 0x000002B95FD35850>
myGen = myGenerator()
print(next(myGen), end=" ")
print("Hello From Python")
print(next(myGen), end=" ")
1 Hello From Python
2
for number in myGen:
print(number)
3
4
083 - Decorators – Intro#
[1] Sometimes Called Meta Programming
[2] Everything in Python is Object Even Functions
[3] Decorator Take A Function and Add Some Functionality and Return It
[4] Decorator Wrap Other Function and Enhance Their Behaviour
[5] Decorator is Higher Order Function (Function Accept Function As Parameter)
def myDecorator(func): # Decorator
def nestedfunc(): # Any Name Its Just For Decoration
print("Before") # Message From Decorator
func() # Execute Function
print("After") # Message From Decorator
return nestedfunc # Return All Data
def sayHello():
print("Hello from sayHello function")
afterDecoration = myDecorator(sayHello)
afterDecoration()
Before
Hello from sayHello function
After
def myDecorator(func): # Decorator
def nestedfunc(): # Any Name Its Just For Decoration
print("Before") # Message From Decorator
func() # Execute Function
print("After") # Message From Decorator
return nestedfunc # Return All Data
@myDecorator
def sayHello():
print("Hello from sayHello function")
sayHello()
Before
Hello from sayHello function
After
def myDecorator(func): # Decorator
def nestedfunc(): # Any Name Its Just For Decoration
print("Before") # Message From Decorator
func() # Execute Function
print("After") # Message From Decorator
return nestedfunc # Return All Data
@myDecorator
def sayHello():
print("Hello from sayHello function")
def sayHowAreYou():
print("Hello From Say How Are You Function")
sayHello()
sayHowAreYou()
Before
Hello from sayHello function
After
Hello From Say How Are You Function
084 - Decorators – Function with Parameters#
def myDecorator(func): # Decorator
def nestedFunc(num1, num2): # Any Name Its Just For Decoration
if num1 < 0 or num2 < 0:
print("Beware One Of The Numbers Is Less Than Zero")
func(num1, num2) # Execute Function
return nestedFunc # Return All Data
@myDecorator
def calculate(n1, n2):
print(n1 + n2)
calculate(10, 90)
calculate(-5, 90)
100
Beware One Of The Numbers Is Less Than Zero
85
def myDecorator(func): # Decorator
def nestedFunc(num1, num2): # Any Name Its Just For Decoration
if num1 < 0 or num2 < 0:
print("Beware One Of The Numbers Is Less Than Zero")
func(num1, num2) # Execute Function
return nestedFunc # Return All Data
def myDecoratorTwo(func): # Decorator
def nestedFunc(num1, num2): # Any Name Its Just For Decoration
print("Coming From Decorator Two")
func(num1, num2) # Execute Function
return nestedFunc # Return All Data
@myDecorator
@myDecoratorTwo
def calculate(n1, n2):
print(n1 + n2)
calculate(-5, 90)
Beware One Of The Numbers Is Less Than Zero
Coming From Decorator Two
85
@myDecoratorTwo
@myDecorator
def calculate(n1, n2):
print(n1 + n2)
calculate(-5, 90)
Coming From Decorator Two
Beware One Of The Numbers Is Less Than Zero
85
085 - Decorators – Practical Speed Test#
def myDecorator(func): # Decorator
def nestedFunc(*numbers): # Any Name Its Just For Decoration
for number in numbers:
if number < 0:
print("Beware One Of The Numbers Is Less Than Zero")
func(*numbers) # Execute Function
return nestedFunc # Return All Data
@myDecorator
def calculate(n1, n2, n3, n4):
print(n1 + n2 + n3 + n4)
calculate(-5, 90, 50, 150)
Beware One Of The Numbers Is Less Than Zero
285
from time import time
def speedTest(func):
def wrapper():
start = time()
func()
end = time()
print(f"Function Running Time Is: {end - start}")
return wrapper
@speedTest
def bigLoop():
for _ in range(1, 200000):
print("", end="")
bigLoop()
Function Running Time Is: 0.5086829662322998
Week 12#
086 – Practical Loop On Many Iterators With Zip#
zip() Return A Zip Object Contains All Objects
zip() Length Is The Length of Lowest Object
list1 = [1, 2, 3, 4, 5]
list2 = ["A", 'B']
ultimateList = zip(list1, list2)
print(ultimateList)
for item in ultimateList:
print(item) # length of lowest object
<zip object at 0x000002B95FD55A00>
(1, 'A')
(2, 'B')
list1 = [1, 2, 3, 4, 5]
list2 = ["A", 'B']
tuple1 = ("Man", "Woman", "Girl", "Boy")
dict1 = {"Name": "Osama", "Age": 36, "Country": "Egypt", "Skill": "Python"}
for item1, item2 in zip(list1, list2):
print("list1 item =>", item1)
print("list2 item =>", item2)
list1 item => 1
list2 item => A
list1 item => 2
list2 item => B
for item1, item2, item3 in zip(list1, list2, tuple1):
print("list1 item =>", item1)
print("list2 item =>", item2)
print("tuple1 item =>", item3)
list1 item => 1
list2 item => A
tuple1 item => Man
list1 item => 2
list2 item => B
tuple1 item => Woman
for item1, item2, item3, item4 in zip(list1, list2, tuple1, dict1):
print("list1 item =>", item1)
print("list2 item =>", item2)
print("tuple1 item =>", item3)
print("dict1 key =>", item4, "value =>", dict1[item4])
list1 item => 1
list2 item => A
tuple1 item => Man
dict1 key => Name value => Osama
list1 item => 2
list2 item => B
tuple1 item => Woman
dict1 key => Age value => 36
087 - Practical Image Manipulation With Pillow#
pip install pillow
from PIL import Image
myImage = Image.open("picture.jpg")
from IPython.display import display
# myImage.show()
display(myImage)
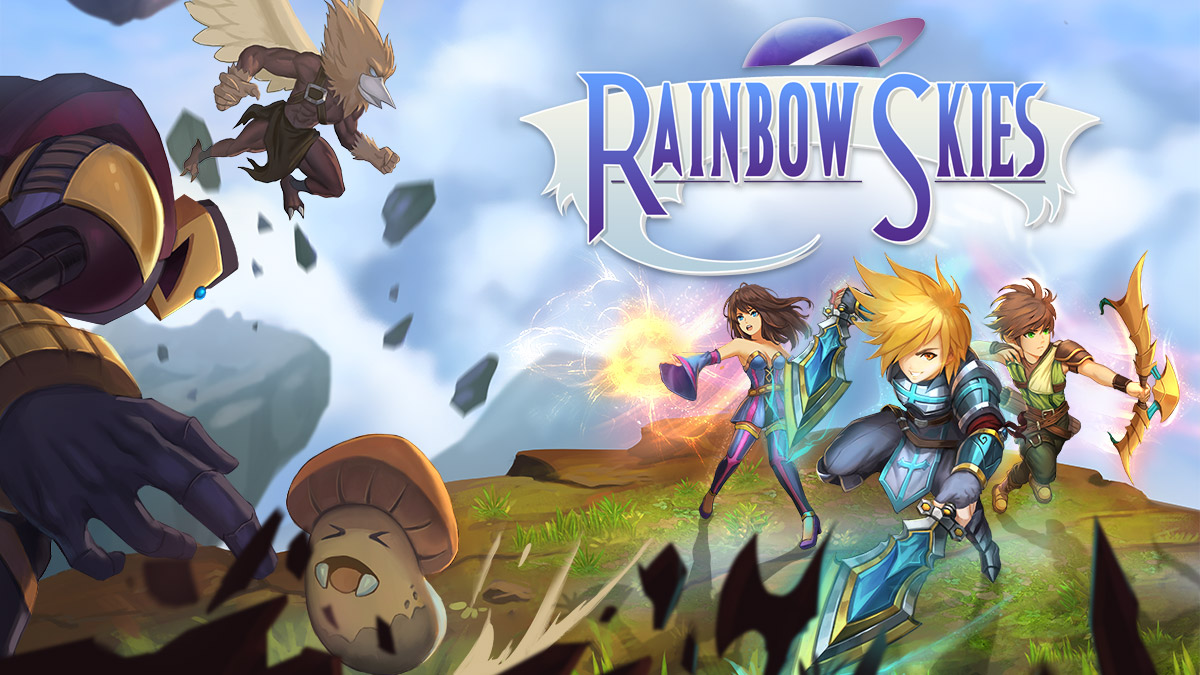
# my cropped image
myBox = (0, 0, 400, 400)
myNewImage = myImage.crop(myBox)
display(myNewImage)
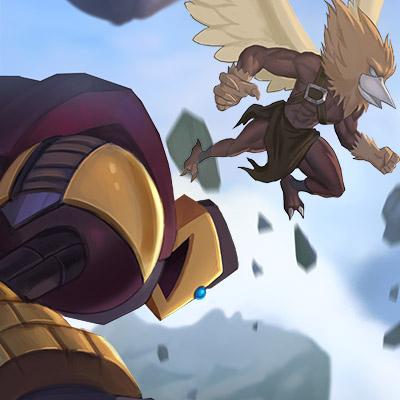
myConverted = myImage.convert("L")
display(myConverted)
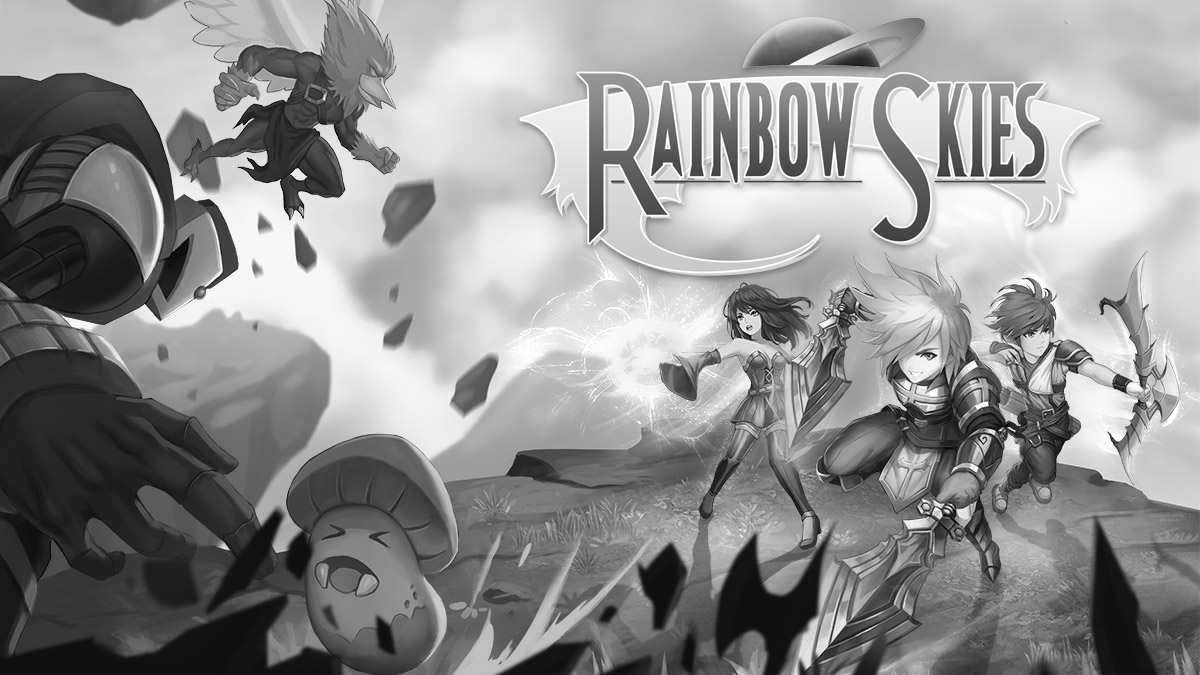
088 - Doc String & Commenting vs Documenting#
[1] Documentation String For Class, Module or Function
[2] Can Be Accessed From The Help and Doc Attributes
[3] Made For Understanding The Functionality of The Complex Code
[4] Theres One Line and Multiple Line Doc Strings
def elzero_function(name):
"""
Elzero Function
It Say Hello From Elzero
Parameter:
name => Person Name That Use Function
Return:
Return Hello Message To The Person
"""
print(f"Hello {name} From Elzero")
elzero_function("Ahmed")
Hello Ahmed From Elzero
import textwrap
output = '\n'.join(dir(elzero_function))
print(textwrap.fill(output, width=80))
__annotations__ __builtins__ __call__ __class__ __closure__ __code__
__defaults__ __delattr__ __dict__ __dir__ __doc__ __eq__ __format__ __ge__
__get__ __getattribute__ __getstate__ __globals__ __gt__ __hash__ __init__
__init_subclass__ __kwdefaults__ __le__ __lt__ __module__ __name__ __ne__
__new__ __qualname__ __reduce__ __reduce_ex__ __repr__ __setattr__ __sizeof__
__str__ __subclasshook__
print(elzero_function.__doc__)
Elzero Function
It Say Hello From Elzero
Parameter:
name => Person Name That Use Function
Return:
Return Hello Message To The Person
help(elzero_function)
Help on function elzero_function in module __main__:
elzero_function(name)
Elzero Function
It Say Hello From Elzero
Parameter:
name => Person Name That Use Function
Return:
Return Hello Message To The Person
089 - Installing And Use Pylint For Better Code#
pip install pylint
def hello():
print("yay")
def say_hello(name):
'''This Function Only Say Hello To Someone'''
msg = "Hello"
return f"{msg} {name}"
090 – Errors And Exceptions Raising#
[1] Exceptions Is A Runtime Error Reporting Mechanism
[2] Exception Gives You The Message To Understand The Problem
[3] Traceback Gives You The Line To Look For The Code in This Line
[4] Exceptions Have Types (SyntaxError, IndexError, KeyError, Etc…)
[5] Exceptions List https://docs.python.org/3/library/exceptions.html
[6] raise Keyword Used To Raise Your Own Exceptions
x = 10
if x < 0:
raise Exception(f"The Number {x} Is Less Than Zero")
else:
print(f"{x} is good number and ok")
print("message after if condition")
10 is good number and ok
message after if condition
y = 10
if type(y) != int:
raise ValueError("Only Numbers Allowed")
print('Print Message After If Condition')
Print Message After If Condition
091 - Exceptions Handling Try, Except, Else, Finally#
Try => Test The Code For Errors
Except => Handle The Errors
Else => If No Errors
Finally => Run The Code
try:
number = int('hi')
except:
print("this is not an integer")
else:
print("this is int")
this is not an integer
try:
number = int(5)
except:
print("this is not an integer")
else:
print("this is int")
this is int
try:
number = int(5)
print("this is int")
except:
print("this is not an integer")
this is int
try:
number = int(5)
print("this is int")
except:
print("this is not an integer")
finally:
print("end")
this is int
end
try:
print(int("hi"))
except ZeroDivisionError:
print("cant divide")
except NameError:
print("identifer not found")
except:
print("error happened")
error happened
try:
print(10/0)
except ZeroDivisionError:
print("cant divide by zero")
except NameError:
print("identifer not found")
except:
print("error happened")
cant divide by zero
092 - Exceptions Handling Advanced Example#
the_file = None
the_tries = 3
while the_tries > 0:
try: # Try To Open The File
print("Enter The File Name With Absolute Path To Open")
print(f"You Have {the_tries} Tries Left")
print("Example: D:\Python\Files\yourfile.extension")
file_name_and_path = input("File Name => : ").strip()
the_file = open(file_name_and_path, 'r')
print(the_file.read())
break
except FileNotFoundError:
print("File Not Found Please Be Sure The Name is Valid")
the_tries -= 1
except:
print("Error Happen")
finally:
if the_file is not None:
the_file.close()
print("File Closed.")
else:
print("All Tries are Done")
Enter The File Name With Absolute Path To Open
You Have 3 Tries Left
Example: D:\Python\Files\yourfile.extension
File Not Found Please Be Sure The Name is Valid
Enter The File Name With Absolute Path To Open
You Have 2 Tries Left
Example: D:\Python\Files\yourfile.extension
File Not Found Please Be Sure The Name is Valid
Enter The File Name With Absolute Path To Open
You Have 1 Tries Left
Example: D:\Python\Files\yourfile.extension
File Not Found Please Be Sure The Name is Valid
All Tries are Done
093 - Debugging Code#
my_list = [1, 2, 3]
my_dictionary = {"Name": "Osama", "Age": 36, "Country": "Egypt"}
for num in my_list:
print(num)
for key, value in my_dictionary.items():
print(f"{key} => {value}")
def function_one_one():
print("Hello From Function One")
function_one_one()
1
2
3
Name => Osama
Age => 36
Country => Egypt
Hello From Function One
094 - Type Hinting#
def say_hello(name) -> str:
print(f"Hello {name}")
say_hello("Ahmed")
Hello Ahmed
def calculate(n1, n2) -> int:
print(n1 + n2)
calculate(10, 40)
50
Week 13#
095 - Regular Expressions Part 1 Intro#
[1] Sequence of Characters That Define A Search Pattern
[2] Regular Expression is Not In Python Its General Concept
[3] Used In [Credit Card Validation, IP Address Validation, Email Validation]
[4] Test RegEx “https://pythex.org/”
[5] Characters Sheet “https://www.debuggex.com/cheatsheet/regex/python”
import re
text = """Hi i am ahmed trying my luck
My Number is 123456789
My Email is Ahmed@Ahmed.com
!@#$%^&*()
123 123
"""
print(re.findall(r'\d' ,text)) #digit
['1', '2', '3', '4', '5', '6', '7', '8', '9', '1', '2', '3', '1', '2', '3']
import textwrap
output = '\n'.join(re.findall(r'\D' ,text)) #not digit
print(textwrap.fill(output, width=80))
H i i a m a h m e d t r y i n g m y l u c k M y N u m b e r i
s M y E m a i l i s A h m e d @ A h m e d . c o m ! @ # $ % ^ & * (
)
output = '\n'.join(re.findall(r'\w' ,text)) #digit and letter
print(textwrap.fill(output, width=80))
H i i a m a h m e d t r y i n g m y l u c k M y N u m b e r i s 1 2 3 4 5 6 7 8
9 M y E m a i l i s A h m e d A h m e d c o m 1 2 3 1 2 3
output = '\n'.join(re.findall(r'\W', text)) # not digit nor letter
print(textwrap.fill(output, width=80))
@ . ! @ # $ % ^ & * ( )
output = '\n'.join(re.findall(r'\d\d', text)) # two consecutive digits
print(textwrap.fill(output, width=80))
12 34 56 78 12 12
output = '\n'.join(re.findall(r'\d\d\d', text)) # three consecutive digits
print(textwrap.fill(output, width=80))
123 456 789 123 123
output = '\n'.join(re.findall(r'\d\s', text)) # digit followed by space
print(textwrap.fill(output, width=80))
9 3 3
output = '\n'.join(re.findall(r'\w\w', text)) # two consecutive digits or two consecutive letters
print(textwrap.fill(output, width=80))
Hi am ah me tr yi ng my lu ck My Nu mb er is 12 34 56 78 My Em ai is Ah me Ah me
co 12 12
output = '\n'.join(re.findall(r'\w\w\w\w\w\w', text))
print(textwrap.fill(output, width=80))
trying Number 123456
output = '\n'.join(re.findall(r'.',text)) # anything except new line
print(textwrap.fill(output, width=80))
H i i a m a h m e d t r y i n g m y l u c k M y N u m b e r i s
1 2 3 4 5 6 7 8 9 M y E m a i l i s A h m e d @ A h m e d . c o m ! @ # $
% ^ & * ( ) 1 2 3 1 2 3
096 - Regular Expressions Part 2 Quantifiers#
* 0 or more
+ 1 or more
? 0 or 1
{2} Exactly 2
{2, 5} Between 2 and 5
{2,} 2 or more
(,5} Up to 5
Explain RegEx “https://regex101.com/”
import re
text = """A
ABC
123 123 1234
ABCD ABCDE
A B C D E F
"""
output = '\n'.join(re.findall(r'A', text)) #find letter A
print(textwrap.fill(output, width=80))
A A A A A
output = '\n'.join(re.findall(r'A\w\w', text))
print(textwrap.fill(output, width=80))
ABC ABC ABC
output = '\n'.join(re.findall(r'A\w\w\w\w', text))
print(textwrap.fill(output, width=80))
ABCDE
output = '\n'.join(re.findall(r'A\w\w\wE', text))
print(textwrap.fill(output, width=80))
ABCDE
output = '\n'.join(re.findall(r'\w\w\w\w', text))
print(textwrap.fill(output, width=80))
1234 ABCD ABCD
output = '\n'.join(re.findall(r'\sD', text)) #space followed by D
print(textwrap.fill(output, width=80))
D
output = '\n'.join(re.findall(r'\w+', text)) #one or more
print(textwrap.fill(output, width=80))
A ABC 123 123 1234 ABCD ABCDE A B C D E F
output = '\n'.join(re.findall(r'\w?', text)) # zero or one
print(textwrap.fill(output, width=80))
A A B C 1 2 3 1 2 3 1 2 3 4 A B C D A B C D E A B C D E F
output = '\n'.join(re.findall(r'\w{3}', text)) # 3 consecutive characters
print(textwrap.fill(output, width=80))
ABC 123 123 123 ABC ABC
output = '\n'.join(re.findall(r'\w{2,4}', text))
print(textwrap.fill(output, width=80))
ABC 123 123 1234 ABCD ABCD
output = '\n'.join(re.findall(r'\w{3,}', text)) #3 to infinity
print(textwrap.fill(output, width=80))
ABC 123 123 1234 ABCD ABCDE
output = '\n'.join(re.findall(r'\w{,3}', text))
print(textwrap.fill(output, width=80))
A ABC 123 123 123 4 ABC D ABC DE A B C D E F
phone = """011 5456-820
Hello
My Name is Osama
12345678
"""
output = '\n'.join(re.findall(r'\d{3}\s\d{4}-\d{3}', phone))
print(textwrap.fill(output, width=80))
011 5456-820
097 - Regular Expressions Part 3 Characters Classes Training’s#
[0-9]
[^0-9]
[A-Z]
[^A-Z]
[a-z]
[^a-z]
text = """011 5456-820
Hello
My Name is Osama
12345678
012 1234-156
!@#$%^&*()_+
a r d
"""
output = '\n'.join(re.findall(r'a r d', text))
print(textwrap.fill(output, width=80))
a r d
output = '\n'.join(re.findall(r'[ard]', text))
print(textwrap.fill(output, width=80))
a a a a r d
output = '\n'.join(re.findall(r'[a-z]', text))
print(textwrap.fill(output, width=80))
e l l o y a m e i s s a m a a r d
output = '\n'.join(re.findall(r'[A-Z]', text))
print(textwrap.fill(output, width=80))
H M N O
output = '\n'.join(re.findall(r'[a-zA-Z0-9]', text))
print(textwrap.fill(output, width=80))
0 1 1 5 4 5 6 8 2 0 H e l l o M y N a m e i s O s a m a 1 2 3 4 5 6 7 8 0 1 2 1
2 3 4 1 5 6 a r d
output = '\n'.join(re.findall(r'[a-m]', text))
print(textwrap.fill(output, width=80))
e l l a m e i a m a a d
output = '\n'.join(re.findall(r'[^a-z]', text)) #not a to z
print(textwrap.fill(output, width=80))
0 1 1 5 4 5 6 - 8 2 0 H M N O 1 2 3 4 5 6 7 8 0 1 2 1 2 3 4 -
1 5 6 ! @ # $ % ^ & * ( ) _ +
output = '\n'.join(re.findall(r'N[a-z]', text))
print(textwrap.fill(output, width=80))
Na
test = " IM 001 yay"
output = '\n'.join(re.findall(r'\s[A-Z]{2}\s[0-9]{3}\s\w{,6}', test))
print(textwrap.fill(output, width=80))
IM 001 yay
098 - Regular Expressions Part 4 Assertions and Email Pattern#
^ Start of String
$ End of string
Match Email
[A-z0-9.]+@[A-z0-9]+.[A-z]+
^[A-z0-9.]+@[A-z0-9]+.(com|net|org|info)$
text = '''012 1234 567
=>012 1234-654
012 1234 567=>
012 1234-654=>
012 1234-654
'''
print(re.findall(r'\d{3}\s\d{4}-?\s?\d{3}', text))
['012 1234 567', '012 1234-654', '012 1234 567', '012 1234-654', '012 1234-654']
print(re.findall(r'^\d{3}\s\d{4}-?\s?\d{3}', text))
['012 1234 567']
email = """osama.elzero@osama.com
kamal@mail.ru"""
print(re.findall(r'[A-z0-9\.]+@[A-z0-9]+\.[A-z]+', email))
['osama.elzero@osama.com', 'kamal@mail.ru']
print(re.search(r'[A-z0-9\.]+@[A-z0-9]+\.(com|net)', email))
<re.Match object; span=(0, 22), match='osama.elzero@osama.com'>
099 - Regular Expressions Part 5 Logical Or And Escaping#
| Or
\ Escape Special Characters
() Separate Groups
import re
text ="""1-HTML
2-CSS
3-PHP
1)HTML
2)CSS
3)PHP
1>HTML
2>CSS
3>PHP
"""
print(re.findall(r'(\d-)(\w+)', text))
[('1-', 'HTML'), ('2-', 'CSS'), ('3-', 'PHP')]
print(re.findall(r'(\d-|\d\))(\w+)', text))
[('1-', 'HTML'), ('2-', 'CSS'), ('3-', 'PHP'), ('1)', 'HTML'), ('2)', 'CSS'), ('3)', 'PHP')]
print(re.findall(r'(\d-|\d\)|\d> )(\w+)', text))
[('1-', 'HTML'), ('2-', 'CSS'), ('3-', 'PHP'), ('1)', 'HTML'), ('2)', 'CSS'), ('3)', 'PHP')]
text= '''012 4578 213
011 4568 (345)
'''
print(re.findall(r'(\d{3}) (\d{4}) (\d{3}|\(\d{3}\))',text))
[('012', '4578', '213'), ('011', '4568', '(345)')]
web = '''http://www.elzero.net
http://elzero.org
https://elzerocourses.com
Hello Osama Elzero'''
print(re.findall(r'(https?://)(www\.)?(\w+\.)(net|org|com)',web))
[('http://', 'www.', 'elzero.', 'net'), ('http://', '', 'elzero.', 'org'), ('https://', '', 'elzerocourses.', 'com')]
100 – Regular Expression Part 6 – Re Module Search And FindAll#
search() => Search A String For A Match And Return A First Match Only
findall() => Returns A List Of All Matches and Empty List if No Match
import re
my_search = re.search("[A-Z]","OsamaElzero")
print(my_search)
<re.Match object; span=(0, 1), match='O'>
import textwrap
output = '\n'.join(dir(my_search))
print(textwrap.fill(output, width=80))
__class__ __class_getitem__ __copy__ __deepcopy__ __delattr__ __dir__ __doc__
__eq__ __format__ __ge__ __getattribute__ __getitem__ __getstate__ __gt__
__hash__ __init__ __init_subclass__ __le__ __lt__ __module__ __ne__ __new__
__reduce__ __reduce_ex__ __repr__ __setattr__ __sizeof__ __str__
__subclasshook__ end endpos expand group groupdict groups lastgroup lastindex
pos re regs span start string
my_search = re.search("[A-Z]{2}","OSamaElzero")
print(my_search.span())
print(my_search.string)
print(my_search.group())
(0, 2)
OSamaElzero
OS
is_email = re.search(r"[A-z0-9\.]+@[A-z0-9]+\.(com|net)","os@osama.com")
if is_email:
print("this is a valid mail")
else:
print("invalid email")
print(is_email.span())
print(is_email.string)
print(is_email.group())
this is a valid mail
(0, 12)
os@osama.com
os@osama.com
email_input = "ahmed@mail.com"
search = re.findall(r"[A-z0-9\.]+@[A-z0-9]+\.com|net", email_input)
empty_list = []
if search != []:
empty_list.append(search)
print("Email Added")
else:
print("Invalid Email")
for email in empty_list:
print(email)
Email Added
['ahmed@mail.com']
101 - Regular Expressions Part 7 Re Module Split And Sub#
split(Pattern, String, MaxSplit) => Return A List Of Elements Splitted On Each Match
sub(Pattern, Replace, String, ReplaceCount) => Replace Matches With What You Want
import re
string_one = "I Love Python"
search_one = re.split(r"\s",string_one)
searchOne= re.split(r"\s",string_one,1)
print(search_one)
print(searchOne)
['I', 'Love', 'Python']
['I', 'Love Python']
string_two = "How-To_Write_A_Very-Good-Article"
search_two = re.split(r"-|_", string_two)
print(search_two)
['How', 'To', 'Write', 'A', 'Very', 'Good', 'Article']
for word in search_two:
print(word)
How
To
Write
A
Very
Good
Article
for counter, word in enumerate(search_two, 1):
if len(word) == 1:
continue
print(f"Word Number: {counter} => {word.lower()}")
Word Number: 1 => how
Word Number: 2 => to
Word Number: 3 => write
Word Number: 5 => very
Word Number: 6 => good
Word Number: 7 => article
my_string = "I Love Python"
print(re.sub(r"\s", "-", my_string,))
print(re.sub(r"\s", "-", my_string, 1))
I-Love-Python
I-Love Python
102 - Regular Expressions Part 8 Group Trainings And Flags#
import re
my_web = "https://www.elzero.org:8080/category.php?article=105?name=how-to-do"
search = re.search(r"(https?)://(www)?\.?(\w+)\.(\w+):?(\d+)?/?(.+)", my_web,re.MULTILINE)
print(search.group())
print(search.groups())
https://www.elzero.org:8080/category.php?article=105?name=how-to-do
('https', 'www', 'elzero', 'org', '8080', 'category.php?article=105?name=how-to-do')
for group in search.groups():
print(group)
https
www
elzero
org
8080
category.php?article=105?name=how-to-do
print(f"Protocol: {search.group(1)}")
print(f"Sub Domain: {search.group(2)}")
print(f"Domain Name: {search.group(3)}")
print(f"Top Level Domain: {search.group(4)}")
print(f"Port: {search.group(5)}")
print(f"Query String: {search.group(6)}")
Protocol: https
Sub Domain: www
Domain Name: elzero
Top Level Domain: org
Port: 8080
Query String: category.php?article=105?name=how-to-do
Week 14#
103 – OOP – Part 1 – Introduction#
Python Support Object Oriented Programming
OOP Is A Paradigm Or Coding Style
OOP Paradigm = >
Means Structuring Program So The Methods[Functions] and Attributes[Data] Are Bundled Into ObjectsMethods = > Act As Function That Use The Information Of The Object
Python Is Multi-Paradigm Programming Language[Procedural, OOP, Functional]
Procedural = > Structure App Like Recipe, Sets Of Steps To Make The Task
Functional = > Built On the Concept of Mathematical Functions
OOP Allow You To Organize Your Code and Make It Readable and Reusable
Everything in Python is Object
If Man Is Object
Attributes = > Name, Age, Address, Phone Number, Info[Can Be Differnet]
Methods[Behaviors] = > Walking, Eating, Singing, Playing
If Car Is Object
Attributes = > Model, Colour, Price
Methods[Behaviors] = > Walking, Stopping
Class Is The Template For Creating Objects[Object Constructor | Blueprint]
104 – OOP – Part 2 – Class Syntax And Information#
[01] Class is The Blueprint Or Construtor Of The Object
[02] Class Instantiate Means Create Instance of A Class
[03] Instance => Object Created From Class And Have Their Methods and Attributes
[04] Class Defined With Keyword class
[05] Class Name Written With PascalCase [UpperCamelCase] Style
[06] Class May Contains Methods and Attributes
[07] When Creating Object Python Look For The Built In init Method
[08] init Method Called Every Time You Create Object From Class
[09] init Method Is Initialize The Data For The Object
[10] Any Method With Two Underscore in The Start and End Called Dunder or Magic Method
[11] self Refer To The Current Instance Created From The Class And Must Be First Param
[12] self Can Be Named Anything
[13] In Python You Dont Need To Call new() Keyword To Create Object
Syntax
class Name:
Constructor => Do Instantiation [ Create Instance From A Class ]
Each Instance Is Separate Object
def __init__(self, other_data)
Body Of Function
class Member:
def __init__(self):
print("a new member has been added")
Member()
Member()
a new member has been added
a new member has been added
<__main__.Member at 0x2b9603ca450>
import textwrap
output = '\n'.join(dir(Member))
print(textwrap.fill(output, width=80))
__class__ __delattr__ __dict__ __dir__ __doc__ __eq__ __format__ __ge__
__getattribute__ __getstate__ __gt__ __hash__ __init__ __init_subclass__ __le__
__lt__ __module__ __ne__ __new__ __reduce__ __reduce_ex__ __repr__ __setattr__
__sizeof__ __str__ __subclasshook__ __weakref__
output = '\n'.join(dir(str))
print(textwrap.fill(output, width=80))
__add__ __class__ __contains__ __delattr__ __dir__ __doc__ __eq__ __format__
__ge__ __getattribute__ __getitem__ __getnewargs__ __getstate__ __gt__ __hash__
__init__ __init_subclass__ __iter__ __le__ __len__ __lt__ __mod__ __mul__ __ne__
__new__ __reduce__ __reduce_ex__ __repr__ __rmod__ __rmul__ __setattr__
__sizeof__ __str__ __subclasshook__ capitalize casefold center count encode
endswith expandtabs find format format_map index isalnum isalpha isascii
isdecimal isdigit isidentifier islower isnumeric isprintable isspace istitle
isupper join ljust lower lstrip maketrans partition removeprefix removesuffix
replace rfind rindex rjust rpartition rsplit rstrip split splitlines startswith
strip swapcase title translate upper zfill
member_one = Member()
member_two = Member()
print(member_one.__class__)
a new member has been added
a new member has been added
<class '__main__.Member'>
105 – OOP – Part 3 – Instance Attributes And Methods Part 1#
Self: Point To Instance Created From Class
Instance Attributes: Instance Attributes Defined Inside The Constructor
Instance Methods: Take Self Parameter Which Point To Instance Created From Class
Instance Methods Can Have More Than One Parameter Like Any Function
Instance Methods Can Freely Access Attributes And Methods On The Same Object
Instance Methods Can Access The Class Itself
class Member:
def __init__(self):
self.name = "Osama"
member_one = Member()
member_two = Member()
output = '\n'.join(dir(Member))
print(textwrap.fill(output, width=80))
__class__ __delattr__ __dict__ __dir__ __doc__ __eq__ __format__ __ge__
__getattribute__ __getstate__ __gt__ __hash__ __init__ __init_subclass__ __le__
__lt__ __module__ __ne__ __new__ __reduce__ __reduce_ex__ __repr__ __setattr__
__sizeof__ __str__ __subclasshook__ __weakref__
print(member_one.name)
print(member_two.name)
Osama
Osama
class Member:
def __init__(self, first_name):
self.name = first_name
member_one = Member("osama")
member_two = Member("ahmed")
print(member_one.name)
print(member_two.name)
osama
ahmed
class Member:
def __init__(self, first_name, middle_name, last_name):
self.fname = first_name
self.mname = middle_name
self.lname = last_name
member_one = Member("osama","mohamed","elsayed")
member_two = Member("ahmed","hany","darwish")
print(member_one.fname, member_one.mname, member_one.lname)
print(member_two.fname)
osama mohamed elsayed
ahmed
106 – OOP – Part 4 – Instance Attributes And Methods Part 2#
Self: Point To Instance Created From Class
Instance Attributes: Instance Attributes Defined Inside The Constructor
Instance Methods: Take Self Parameter Which Point To Instance Created From Class
Instance Methods Can Have More Than One Parameter Like Any Function
Instance Methods Can Freely Access Attributes And Methods On The Same Object
Instance Methods Can Access The Class Itself
class Member:
def __init__(self, first_name, middle_name, last_name):
self.fname = first_name
self.mname = middle_name
self.lname = last_name
def full_name(self):
return f"{self.fname} {self.mname} {self.lname}"
def name_with_title(self):
return f"Hello Mr {self.fname}"
member_one = Member("Osama", "Mohamed", "Elsayed")
member_two = Member("Ahmed", "Ali", "Mahmoud")
print(member_one.full_name())
print(member_one.name_with_title())
Osama Mohamed Elsayed
Hello Mr Osama
class Member:
def __init__(self, first_name, middle_name, last_name, gender):
self.fname = first_name
self.mname = middle_name
self.lname = last_name
self.gender = gender
def full_name(self):
return f"{self.fname} {self.mname} {self.lname}"
def name_with_title(self):
if self.gender == "Male":
return f"Hello Mr {self.fname}"
elif self.gender == "Female":
return f"Hello Miss {self.fname}"
else:
return f"Hello {self.fname}"
def get_all_info(self):
return f"{self.name_with_title()}, Your Full Name Is: {self.full_name()}"
member_one = Member("Osama", "Mohamed", "Elsayed", "Male")
member_two = Member("Ahmed", "Hany", "Darwish", "Male")
print(member_one.get_all_info())
Hello Mr Osama, Your Full Name Is: Osama Mohamed Elsayed
107 - OOP – Part 5 Class Attributes#
Class Attributes: Attributes Defined Outside The Constructor
class Member:
not_allowed_names = ["Hell", "Shit", "Baloot"]
users_num = 0
def __init__(self, first_name, middle_name, last_name, gender):
self.fname = first_name
self.mname = middle_name
self.lname = last_name
self.gender = gender
Member.users_num += 1 # Member.users_num = Member.users_num + 1
def full_name(self):
if self.fname in Member.not_allowed_names:
raise ValueError("Name Not Allowed")
else:
return f"{self.fname} {self.mname} {self.lname}"
def name_with_title(self):
if self.gender == "Male":
return f"Hello Mr {self.fname}"
elif self.gender == "Female":
return f"Hello Miss {self.fname}"
else:
return f"Hello {self.fname}"
def get_all_info(self):
return f"{self.name_with_title()}, Your Full Name Is: {self.full_name()}"
def delete_user(self):
Member.users_num -= 1 # Member.users_num = Member.users_num -1
return f"User {self.fname} Is Deleted."
output = '\n'.join(dir(Member))
print(textwrap.fill(output, width=80))
__class__ __delattr__ __dict__ __dir__ __doc__ __eq__ __format__ __ge__
__getattribute__ __getstate__ __gt__ __hash__ __init__ __init_subclass__ __le__
__lt__ __module__ __ne__ __new__ __reduce__ __reduce_ex__ __repr__ __setattr__
__sizeof__ __str__ __subclasshook__ __weakref__ delete_user full_name
get_all_info name_with_title not_allowed_names users_num
print(Member.users_num)
member_one = Member("Osama", "Mohamed", "Elsayed", "Male")
member_two = Member("Ahmed", "Ali", "Mahmoud", "Male")
member_three = Member("Mona", "Ali", "Mahmoud", "Female")
member_four = Member("Shit", "Hell", "Metal", "DD")
print(Member.users_num)
0
4
print(member_four.delete_user())
print(Member.users_num)
User Shit Is Deleted.
3
output = '\n'.join(dir(member_one))
print(textwrap.fill(output, width=80))
__class__ __delattr__ __dict__ __dir__ __doc__ __eq__ __format__ __ge__
__getattribute__ __getstate__ __gt__ __hash__ __init__ __init_subclass__ __le__
__lt__ __module__ __ne__ __new__ __reduce__ __reduce_ex__ __repr__ __setattr__
__sizeof__ __str__ __subclasshook__ __weakref__ delete_user fname full_name
gender get_all_info lname mname name_with_title not_allowed_names users_num
print(member_one.fname, member_one.mname, member_one.lname)
print(member_two.fname)
print(member_three.fname)
Osama Mohamed Elsayed
Ahmed
Mona
print(member_two.full_name())
print(member_two.name_with_title())
Ahmed Ali Mahmoud
Hello Mr Ahmed
print(member_three.get_all_info())
Hello Miss Mona, Your Full Name Is: Mona Ali Mahmoud
108 - OOP – Part 6 Class Methods and Static Methods#
Class Methods:
Marked With @classmethod Decorator To Flag It As Class Method
It Take Cls Parameter Not Self To Point To The Class not The Instance
It Doesn’t Require Creation of a Class Instance
Used When You Want To Do Something With The Class Itself
Static Methods:
It Takes No Parameters
Its Bound To The Class Not Instance
Used When Doing Something Doesnt Have Access To Object Or Class But Related To Class
class Member:
not_allowed_names = ["Hell", "Shit", "Baloot"]
users_num = 0
@classmethod
def show_users_count(cls):
print(f"we have {cls.users_num} users in our system")
@staticmethod
def say_hello():
print("Hello from static method")
def __init__(self, first_name, middle_name, last_name, gender):
self.fname = first_name
self.mname = middle_name
self.lname = last_name
self.gender = gender
Member.users_num += 1 # Member.users_num = Member.users_num + 1
def full_name(self):
if self.fname in Member.not_allowed_names:
raise ValueError("Name Not Allowed")
else:
return f"{self.fname} {self.mname} {self.lname}"
def name_with_title(self):
if self.gender == "Male":
return f"Hello Mr {self.fname}"
elif self.gender == "Female":
return f"Hello Miss {self.fname}"
else:
return f"Hello {self.fname}"
def get_all_info(self):
return f"{self.name_with_title()}, Your Full Name Is: {self.full_name()}"
def delete_user(self):
Member.users_num -= 1 # Member.users_num = Member.users_num -1
return f"User {self.fname} Is Deleted."
member_one = Member("Osama", "Mohamed", "Elsayed", "Male")
member_two = Member("Ahmed", "Ali", "Mahmoud", "Male")
member_three = Member("Mona", "Ali", "Mahmoud", "Female")
member_four = Member("Shit", "Hell", "Metal", "DD")
Member.show_users_count()
we have 4 users in our system
print(member_one.full_name())
print(Member.full_name(member_one))
Osama Mohamed Elsayed
Osama Mohamed Elsayed
Member.say_hello()
Hello from static method
109 - OOP – Part 7 Class Magic Methods#
Everything in Python is An Object
init Called Automatically When Instantiating Class
self.class The class to which a class instance belongs
str Gives a Human-Readable Output of the Object
len Returns the Length of the Container
Called When We Use the Built-in len() Function on the Object
class Skill:
def __init__(self) -> None:
self.skills = ["HTML","Css","Js"]
profile = Skill()
print(profile)
print(profile.__class__)
<__main__.Skill object at 0x000002B9603DA9D0>
<class '__main__.Skill'>
my_string = "Osama"
print(my_string.__class__)
print(type(my_string))
<class 'str'>
<class 'str'>
output = '\n'.join(dir(str))
print(textwrap.fill(output, width=80))
__add__ __class__ __contains__ __delattr__ __dir__ __doc__ __eq__ __format__
__ge__ __getattribute__ __getitem__ __getnewargs__ __getstate__ __gt__ __hash__
__init__ __init_subclass__ __iter__ __le__ __len__ __lt__ __mod__ __mul__ __ne__
__new__ __reduce__ __reduce_ex__ __repr__ __rmod__ __rmul__ __setattr__
__sizeof__ __str__ __subclasshook__ capitalize casefold center count encode
endswith expandtabs find format format_map index isalnum isalpha isascii
isdecimal isdigit isidentifier islower isnumeric isprintable isspace istitle
isupper join ljust lower lstrip maketrans partition removeprefix removesuffix
replace rfind rindex rjust rpartition rsplit rstrip split splitlines startswith
strip swapcase title translate upper zfill
my_string = 12
print(type(my_string))
print(my_string.__class__)
<class 'int'>
<class 'int'>
my_string = "Osama"
print(str.upper(my_string))
print(my_string.upper())
OSAMA
OSAMA
print(profile)
<__main__.Skill object at 0x000002B9603DA9D0>
class Skill:
def __init__(self) -> None:
self.skills = ["HTML", "Css", "Js"]
def __str__(self) -> str:
return f"this is my skills => {self.skills}"
profile = Skill()
print(profile)
this is my skills => ['HTML', 'Css', 'Js']
print(len(profile)) #error
class Skill:
def __init__(self) -> None:
self.skills = ["HTML", "Css", "Js"]
def __str__(self) -> str:
return f"this is my skills => {self.skills}"
def __len__(self):
return len(self.skills)
profile = Skill()
print(len(profile))
3
profile.skills.append("PHP")
profile.skills.append("MySQL")
print(len(profile))
5
110 - OOP – Part 8 Inheritance#
class Food: #Base class
def __init__(self):
print("Food is created from base class")
def eat(self):
print("Eat method from base class")
class Apple(Food): #Derived class
def __init__(self):
print("Apple is created from derived class")
food_one = Food()
food_two = Apple()
Food is created from base class
Apple is created from derived class
food_two.eat()
Eat method from base class
class Food: #Base class
def __init__(self, name, price):
self.name = name
self.price = price
print(f"{self.name} is created from base class")
def eat(self):
print("Eat method from base class")
food_one = Food("Pizza",150)
Pizza is created from base class
class Apple(Food): # Derived class
def __init__(self, name, price, amount):
# Food.__init__(self,name) #create instance from base class
super().__init__(name, price)
self.amount = amount
print(f"{self.name} Is Created From Derived Class And Price Is {self.price} And Amount Is {self.amount}")
def get_from_tree(self):
print("Get From Tree From Derived Class")
food_two = Apple("pizza",150,500)
pizza is created from base class
pizza Is Created From Derived Class And Price Is 150 And Amount Is 500
food_two.eat()
food_two.get_from_tree()
Eat method from base class
Get From Tree From Derived Class
111 – OOP – Part 9 – Multiple Inheritance And Method Overriding#
class BaseOne:
def __init__(self):
print("Base One")
def func_one(self):
print("One")
class BaseTwo:
def __init__(self):
print("Base Two")
def func_two(self):
print("Two")
class Derived(BaseOne, BaseTwo):
pass
my_var = Derived()
Base One
print(Derived.mro())
[<class '__main__.Derived'>, <class '__main__.BaseOne'>, <class '__main__.BaseTwo'>, <class 'object'>]
print(my_var.func_one)
print(my_var.func_two)
my_var.func_one()
my_var.func_two()
<bound method BaseOne.func_one of <__main__.Derived object at 0x000002B960418D50>>
<bound method BaseTwo.func_two of <__main__.Derived object at 0x000002B960418D50>>
One
Two
DerivedTwo will inherit from base
class Base:
pass
class DerivedOne(Base):
pass
class DerivedTwo(DerivedOne):
pass
112 – OOP – Part 10 – Polymorphism#
n1 = 10
n2 = 20
print(n1 + n2)
30
s1 = "Hello"
s2 = "Python"
print(s1+ " " + s2) # + adds numbers and concatenates strings
HelloPython
print(len([1,2,3,4,5]))
print(len("osama elzero"))
print(len({"Key_One": 1, "Key_Two": 2}))
#len can do multiple tasks based on type
5
12
2
class A:
def do_something(self):
print("From Class A")
raise NotImplementedError("Derived Class Must Implement This Method") #raises error if B doesnt define do_something function
class B(A):
def do_something(self):
print("From Class B")
class C(A):
def do_something(self):
print("From Class C")
my_instance = B()
my_instance.do_something()
From Class B
113 – OOP – Part 11 – Encapsulation#
Encapsulation
Restrict Access To The Data Stored in Attirbutes and Methods Public
Every Attribute and Method That We Used So Far Is Public
Attributes and Methods Can Be Modified and Run From Everywhere
Inside Our Outside The Class Protected
Attributes and Methods Can Be Accessed From Within The Class And Sub Classes
Attributes and Methods Prefixed With One Underscore _ Private
Attributes and Methods Can Be Accessed From Within The Class Or Object Only
Attributes Cannot Be Modified From Outside The Class
Attributes and Methods Prefixed With Two Underscores __
Attributes = Variables = Properties
class Member:
def __init__(self,name) -> None:
self.name = name #public
one = Member("Ahmed")
print(one.name)
one.name = "Sayed"
print(one.name)
Ahmed
Sayed
class Member:
def __init__(self,name) -> None:
self._name = name #protected
one = Member("Ahmed")
print(one._name)
one._name = "Sayed"
print(one._name)
#Shouldnt do that
Ahmed
Sayed
class Member:
def __init__(self,name) -> None:
self.__name = name #protected
one = Member("Ahmed")
print(one.__name)
one.__name = “Sayed”
print(one.__name) #error cuz its protected
class Member:
def __init__(self, name):
self.__name = name # Private
def say_hello(self):
return f"Hello {self.__name}"
one = Member("ahmed")
print(one.say_hello())
Hello ahmed
print(one._Member__name)
#Shouldnt do that
ahmed
114 – OOP – Part 12 – Getters And Setters#
class Member:
def __init__(self, name):
self.__name = name
def say_hello(self):
return f"Hello {self.__name}"
def get_name(self): #Getter
return self.__name
def set_name(self,new_name): # setter
self.__name = new_name
one = Member("Ahmed")
print(one.get_name())
one.set_name("abbas")
print(one.get_name())
Ahmed
abbas
115 – OOP – Part 13 – @Property Decorator#
class Member:
def __init__(self, name, age):
self.name = name
self.age = age
def say_hello(self):
return f"Hello {self.name}"
def age_in_days(self):
return self.age * 365
one = Member("Ahmed", 21)
print(one.name)
print(one.age)
print(one.say_hello())
Ahmed
21
Hello Ahmed
print(one.age_in_days())
print(one.age_in_days)
7665
<bound method Member.age_in_days of <__main__.Member object at 0x000001F0C00C9590>>
class Member:
def __init__(self, name, age):
self.name = name
self.age = age
def say_hello(self):
return f"Hello {self.name}"
@property
def age_in_days(self):
return self.age * 365
one = Member("Ahmed", 21)
print(one.age_in_days)
7665
116 – OOP – Part 14 – ABCs Abstract Base Class#
Class Called Abstract Class If it Has One or More Abstract Methods
abc module in Python Provides Infrastructure for Defining Custom Abstract Base Classes.
By Adding @absttractmethod Decorator on The Methods
ABCMeta Class Is a Metaclass Used For Defining Abstract Base Class
from abc import ABCMeta, abstractmethod
class Programming(metaclass=ABCMeta):
@abstractmethod
def has_oop(self):
pass
class Python(Programming):
def has_oop(self):
return "yes"
class Pascal(Programming):
def has_oop(self):
return "no"
#one = Programming() error
one = Python()
print(one.has_oop())
yes
from abc import ABCMeta, abstractmethod
class Programming(metaclass=ABCMeta):
@abstractmethod
def has_oop(self):
pass
class Python(Programming):
def has_oop(self):
return "yes"
class Pascal(Programming):
pass
# one = Programming() error
#one = Pascal() error
#print(one.has_oop()) error
Week 15#
117 – Databases – Intro To Database#
Database Is A Place Where We Can Store Data
Database Organized Into Tables (Users, Categories)
Tables Has Columns (ID, Username, Password)
There’s Many Types Of Databases (MongoDB, MySQL, SQLite)
SQL Stand For Structured Query Language
SQLite => Can Run in Memory or in A Single File
You Can Browse File With https://sqlitebrowser.org/
Data Inside Database Has Types (Text, Integer, Date)
118 – Databases – SQLite Create Database And Connect#
Connect Execute Close
import sqlite3
# Create Database and connect
db = sqlite3.connect("app.db")
# Create the tables and fields
db.execute("CREATE TABLE IF NOT EXISTS skills (name TEXT, progress INTEGRER, user_id INTEGER)")
<sqlite3.Cursor at 0x1bbefedaac0>
# Close Database
db.close()
119 – Databases – SQLite Insert Data Into Database#
cursor => All Operation in SQL Done By Cursor Not The Connection Itself
commit => Save All Changes
# Import SQLite Module
import sqlite3
# Create Database And Connect
db = sqlite3.connect("app.db")
# Setting Up The Cursor
cr = db.cursor()
# Create The Tables and Fields
cr.execute("create table if not exists users (user_id integer, name text)")
cr.execute(
"create table if not exists skills (name text, progress integer, user_id integer)")
<sqlite3.Cursor at 0x1bbefedb1c0>
# Inserting Data
cr.execute("insert into users(user_id, name) values(1, 'Ahmed')")
cr.execute("insert into users(user_id, name) values(2, 'Sayed')")
cr.execute("insert into users(user_id, name) values(3, 'Osama')")
<sqlite3.Cursor at 0x1bbefedb1c0>
# Save (Commit) Changes
db.commit()
Delete db file before running the for loop
my_list = ["Ahmed", "Sayed", "Mahmoud", "Ali", "Kamel", "Ibrahim", "Enas"]
for key, user in enumerate(my_list):
cr.execute(f"insert into users(user_id, name) values({key + 1}, '{user}')")
db.commit()
#Close Database
db.close()
120 – Databases – SQLite Retrieve Data From Database#
fetchone => returns a single record or None if no more rows are available.
fetchall => fetches all the rows of a query result. It returns all the rows as a list of tuples. An empty list is returned if there is no record to fetch.
fetchmany(size) =>
Delete the database file again
# Import SQLite Module
import sqlite3
# Create Database And Connect
db = sqlite3.connect("app.db")
# Setting Up The Cursor
cr = db.cursor()
# Create The Tables and Fields
cr.execute("create table if not exists users (user_id integer, name text)")
cr.execute("create table if not exists skills (name text, progress integer, user_id integer)")
<sqlite3.Cursor at 0x1bbefedab40>
# Inserting Data
cr.execute("insert into users(user_id, name) values(1, 'Ahmed')")
cr.execute("insert into users(user_id, name) values(2, 'Sayed')")
cr.execute("insert into users(user_id, name) values(3, 'Osama')")
db.commit()
# Fetch Data
cr.execute("select name from users")
print(cr.fetchone())
print(cr.fetchone())
print(cr.fetchone())
print(cr.fetchone())
('Ahmed',)
('Sayed',)
('Osama',)
('Ahmed',)
cr.execute("select user_id, name from users")
#print(cr.fetchall())
print(cr.fetchmany(2))
[(1, 'Ahmed'), (2, 'Sayed')]
121 – Databases – SQLite Training On Everything#
import sqlite3
def get_all_data():
try:
# Connect To Database
db = sqlite3.connect("app.db")
# Print Success Message
print("Connected To Database Successfully")
# Setting Up The Cursor
cr = db.cursor()
# Fetch Data From Database
cr.execute("select * from users")
# Assign Data To Variable
results = cr.fetchall()
# Print Number Of Rows
print(f"Database Has {len(results)} Rows.")
# Printing Message
print("Showing Data:")
# Loop On Results
for row in results:
print(f"UserID => {row[0]},", end=" ")
print(f"Username => {row[1]}")
except sqlite3.Error as er:
print(f"Error Reading Data {er}")
finally:
if (db):
# Close Database Connection
db.close()
print("Connection To Database Is Closed")
get_all_data()
Connected To Database Successfully
Database Has 9 Rows.
Showing Data:
UserID => 1, Username => Ahmed
UserID => 2, Username => Sayed
UserID => 3, Username => Osama
UserID => 1, Username => Ahmed
UserID => 2, Username => Sayed
UserID => 3, Username => Osama
UserID => 1, Username => Ahmed
UserID => 2, Username => Sayed
UserID => 3, Username => Osama
Connection To Database Is Closed
122 – Databases – SQLite Update And Delete From Database#
# Import SQLite Module
import sqlite3
# Create Database And Connect
db = sqlite3.connect("app.db")
# Setting Up The Cursor
cr = db.cursor()
# Create The Tables and Fields
cr.execute("create table if not exists users (user_id integer, name text)")
cr.execute(
"create table if not exists skills (name text, progress integer, user_id integer)")
<sqlite3.Cursor at 0x14e74c94fc0>
# Inserting Data
cr.execute("insert into users(user_id, name) values(1, 'Ahmed')")
cr.execute("insert into users(user_id, name) values(2, 'Sayed')")
cr.execute("insert into users(user_id, name) values(3, 'Osama')")
db.commit()
# Update Data
cr.execute("update users set name = 'Ahmed' where user_id = 1")
<sqlite3.Cursor at 0x14e74c94fc0>
# Delete Data
cr.execute("delete from users where user_id = 4")
<sqlite3.Cursor at 0x14e74c94fc0>
# Fetch Data
cr.execute("select * from users")
print(cr.fetchone())
(1, 'Ahmed')
# Save (Commit) Changes
db.commit()
# Close Database
db.close()
123 – Databases – SQLite Create Skills App Part 1#
# Input Big Message
input_message = """
What Do You Want To Do ?
"s" => Show All Skills
"a" => Add New Skill
"d" => Delete A Skill
"u" => Update Skill Progress
"q" => Quit The App
Choose Option:
"""
# Input Option Choose
user_input = input(input_message).strip().lower()
# Command List
commands_list = ["s", "a", "d", "u", "q"]
# Define The Methods
def show_skills():
print("Show Skills")
def add_skill():
print("Add Skill")
def delete_skill():
print("Delete Skill")
def update_skill():
print("Update Skill Progress")
# Check If Command Is Exists
if user_input in commands_list:
print(f"Command Found {user_input}")
if user_input == "s":
show_skills()
elif user_input == "a":
add_skill()
elif user_input == "d":
delete_skill()
elif user_input == "u":
update_skill()
else:
print("App Is Closed.")
else:
print(f"Sorry This Command \"{user_input}\" Is Not Found")
Command Found u
Update Skill Progress
124 – Databases – SQLite Create Skills App Part 2#
# Import SQLite Module
import sqlite3
# Create Database And Connect
db = sqlite3.connect("skill.db")
# Setting Up The Cursor
cr = db.cursor()
# My User ID
uid = 1
# Commit and Close Method
def commit_and_close():
# Save (Commit) Changes
db.commit()
# Close Database
db.close()
print("Connection To Database Is Closed")
# Input Big Message
input_message = """
What Do You Want To Do ?
"s" => Show All Skills
"a" => Add New Skill
"d" => Delete A Skill
"u" => Update Skill Progress
"q" => Quit The App
Choose Option:
"""
# Input Option Choose
user_input = input(input_message).strip().lower()
# Command List
commands_list = ["s", "a", "d", "u", "q"]
# Define The Methods
def show_skills():
print("Show Skills")
commit_and_close()
def add_skill():
sk = input("Write Skill Name: ").strip().capitalize()
prog = input("Write Skill Progress ").strip()
cr.execute(
f"insert into skills(name, progress, user_id) values('{sk}', '{prog}', '{uid}')")
commit_and_close()
def delete_skill():
sk = input("Write Skill Name: ").strip().capitalize()
cr.execute(f"delete from skills where name = '{sk}' and user_id = '{uid}'")
commit_and_close()
def update_skill():
print("Update Skill Progress")
commit_and_close()
# Check If Command Is Exists
if user_input in commands_list:
# print(f"Command Found {user_input}")
if user_input == "s":
show_skills()
elif user_input == "a":
add_skill()
elif user_input == "d":
delete_skill()
elif user_input == "u":
update_skill()
else:
print("App Is Closed.")
else:
print(f"Sorry This Command \"{user_input}\" Is Not Found")
App Is Closed.
125 – Databases – SQLite Create Skills App Part 3#
# Import SQLite Module
import sqlite3
# Create Database And Connect
db = sqlite3.connect("skill.db")
# Setting Up The Cursor
cr = db.cursor()
def commit_and_close():
"""Commit Changes and Close Connection To Database"""
db.commit() # Save (Commit) Changes
db.close() # Close Database
print("Connection To Database Is Closed")
# My User ID
uid = 1
# Input Big Message
input_message = """
What Do You Want To Do ?
"s" => Show All Skills
"a" => Add New Skill
"d" => Delete A Skill
"u" => Update Skill Progress
"q" => Quit The App
Choose Option:
"""
# Input Option Choose
user_input = input(input_message).strip().lower()
# Command List
commands_list = ["s", "a", "d", "u", "q"]
# Define The Methods
def show_skills():
cr.execute(f"select * from skills where user_id = '{uid}'")
results = cr.fetchall()
print(f"You Have {len(results)} Skills.")
if len(results) > 0:
print("Showing Skills With Progress: ")
for row in results:
print(f"Skill => {row[0]},", end=" ")
print(f"Progress => {row[1]}%")
commit_and_close()
def add_skill():
sk = input("Write Skill Name: ").strip().capitalize()
prog = input("Write Skill Progress ").strip()
cr.execute(
f"insert into skills(name, progress, user_id) values('{sk}', '{prog}', '{uid}')")
commit_and_close()
def delete_skill():
sk = input("Write Skill Name: ").strip().capitalize()
cr.execute(f"delete from skills where name = '{sk}' and user_id = '{uid}'")
commit_and_close()
def update_skill():
print("Update Skill Progress")
commit_and_close()
# Check If Command Is Exists
if user_input in commands_list:
# print(f"Command Found {user_input}")
if user_input == "s":
show_skills()
elif user_input == "a":
add_skill()
elif user_input == "d":
delete_skill()
elif user_input == "u":
update_skill()
else:
print("App Is Closed.")
else:
print(f"Sorry This Command \"{user_input}\" Is Not Found")
App Is Closed.
126 – Databases – SQLite Create Skills App Part 4#
# Import SQLite Module
import sqlite3
# Create Database And Connect
db = sqlite3.connect("skill.db")
# Setting Up The Cursor
cr = db.cursor()
def commit_and_close():
"""Commit Changes and Close Connection To Database"""
db.commit() # Save (Commit) Changes
db.close() # Close Database
print("Connection To Database Is Closed")
# My User ID
uid = 1
# Input Big Message
input_message = """
What Do You Want To Do ?
"s" => Show All Skills
"a" => Add New Skill
"d" => Delete A Skill
"u" => Update Skill Progress
"q" => Quit The App
Choose Option:
"""
# Input Option Choose
user_input = input(input_message).strip().lower()
# Command List
commands_list = ["s", "a", "d", "u", "q"]
# Define The Methods
def show_skills():
cr.execute(f"select * from skills where user_id = '{uid}'")
results = cr.fetchall()
print(f"You Have {len(results)} Skills.")
if len(results) > 0:
print("Showing Skills With Progress: ")
for row in results:
print(f"Skill => {row[0]},", end=" ")
print(f"Progress => {row[1]}%")
commit_and_close()
def add_skill():
sk = input("Write Skill Name: ").strip().capitalize()
cr.execute(
f"select name from skills where name = '{sk}' and user_id = '{uid}'")
results = cr.fetchone()
if results == None: # Theres No Skill With This Name In Database
prog = input("Write Skill Progress ").strip()
cr.execute(
f"insert into skills(name, progress, user_id) values('{sk}', '{prog}', '{uid}')")
else: # Theres A Skill With This Name in Database
print("You Cannot Add It")
commit_and_close()
def delete_skill():
sk = input("Write Skill Name: ").strip().capitalize()
cr.execute(f"delete from skills where name = '{sk}' and user_id = '{uid}'")
commit_and_close()
def update_skill():
sk = input("Write Skill Name: ").strip().capitalize()
prog = input("Write The New Skill Progress ").strip()
cr.execute(
f"update skills set progress = '{prog}' where name = '{sk}' and user_id = '{uid}'")
commit_and_close()
# Check If Command Is Exists
if user_input in commands_list:
# print(f"Command Found {user_input}")
if user_input == "s":
show_skills()
elif user_input == "a":
add_skill()
elif user_input == "d":
delete_skill()
elif user_input == "u":
update_skill()
else:
print("App Is Closed.")
commit_and_close()
else:
print(f"Sorry This Command \"{user_input}\" Is Not Found")
App Is Closed.
Connection To Database Is Closed
127 – Databases – SQLite Very Important Informations#
import sqlite3
db = sqlite3.connect("skill.db")
cr = db.cursor()
my_tuple = ('Pascal', '65', 4)
cr.execute("insert into skills values(?, ?, ?)", my_tuple)
cr.execute("select * from skills where user_id not in(1, 2, 3)")
results = cr.fetchall()
for row in results:
print(f"Skill Name => {row[0]},", end=" ")
print(f"Skill Progress => {row[1]},", end=" ")
print(f"User ID => {row[2]}")
db.commit()
db.close()
Week 16#
128 - Advanced Lessons – “_name_” And “_main_”#
if __name__ == "__main__":
- __name__ => Built In Variable
- "__main__" => Value Of The __name__ Variable
Executions Methods
- Directly => Execute the Python File Using the Command Line.
- From Import => Import The Code From File To Another File
-------------------------------------------------------------
In Some Cases You Want To Know If You Are Using A Module Method As Import Or You Use The Original Python File
-------------------------------------------------------------------------
In Direct Mode Python Assign A Value "__main__"
To The Built In Variable __name__ in The Background
print(__name__)
__main__
129 - Advanced Lessons – Timing Your Code With Timeit#
timeit: - Get Execution Time Of Code By Running 1M Time And Give You Minimal Time
- It Used For Performance By Testing All Functionality
timeit(stmt, setup, timer, number)
timeit(pass, pass, default, 1.000.000) Default Values
stmt: Code You Want To Measure The Execution Time
setup: Setup Done Before The Code Execution (Import Module Or Anything)
timer: The Timer Value
number: How Many Execution That Will Run
import timeit
print(timeit.timeit("'elzero'" * 1000))
0.01984740002080798
name = "Elzero"
print(timeit.timeit("name = 'Elzero'; name * 1000"))
0.2580245001008734
print(timeit.timeit(stmt="random.randint(0, 50)", setup="import random"))
0.6584104000357911
print(timeit.repeat(stmt="random.randint(0, 50)", setup="import random", repeat=4))
[0.6502906000241637, 0.5979669000953436, 0.7503688000142574, 0.5449246000498533]
130 - Advanced Lessons – Add Logging To Your Code#
Print Out To Console Or File
Print Logs Of What Happens
DEBUG
INFO
WARNING
ERROR
CRITICAL
name => Logging Module Give It To The Default Logger.
Basic Config
level => Level of Severity
filename => File Name and Extension
mode => Mode Of The File a => Append
format => Format For The Log Message
import logging
import textwrap
output = '\n'.join(dir(logging))
print(textwrap.fill(output, width=80))
BASIC_FORMAT BufferingFormatter CRITICAL DEBUG ERROR FATAL FileHandler Filter
Filterer Formatter GenericAlias Handler INFO LogRecord Logger LoggerAdapter
Manager NOTSET NullHandler PercentStyle PlaceHolder RootLogger StrFormatStyle
StreamHandler StringTemplateStyle Template WARN WARNING _STYLES _StderrHandler
__all__ __author__ __builtins__ __cached__ __date__ __doc__ __file__ __loader__
__name__ __package__ __path__ __spec__ __status__ __version__ _acquireLock
_addHandlerRef _checkLevel _defaultFormatter _defaultLastResort _handlerList
_handlers _is_internal_frame _levelToName _lock _logRecordFactory _loggerClass
_nameToLevel _register_at_fork_reinit_lock _releaseLock _removeHandlerRef
_showwarning _srcfile _startTime _str_formatter _warnings_showwarning
addLevelName atexit basicConfig captureWarnings collections config critical
currentframe debug disable error exception fatal getLevelName
getLevelNamesMapping getLogRecordFactory getLogger getLoggerClass handlers info
io lastResort log logMultiprocessing logProcesses logThreads makeLogRecord os
raiseExceptions re root setLogRecordFactory setLoggerClass shutdown sys
threading time traceback warn warning warnings weakref
logging.basicConfig(filename="my_app.log",
filemode="a",
format="(%(asctime)s) | %(name)s | %(levelname)s => '%(message)s'",
datefmt="%d - %B - %Y, %H:%M:%S")
my_logger = logging.getLogger("Elzero")
my_logger.warning("This Is Warning Message")
131 - Advanced Lessons – Unit Testing With Unittest#
Test Runner
The Module That Run The Unit Testing (unittest, pytest)
Test Case
Smallest Unit Of Testing
It Use Asserts Methods To Check For Actions And Responses Test Suite
Collection Of Multiple Tests Or Test Cases Test Report
A Full Report Contains The Failure Or Succeed
unittest
Add Tests Into Classes As Methods
Use a Series of Special Assertion Methods https://docs.python.org/3/library/unittest.html
assert 3*8 ==24, "should be 24"
def test_case_one():
assert 5 * 10 == 50, "Should Be 50"
def test_case_two():
assert 5 * 50 == 250, "Should Be 250"
if __name__ == "__main__":
test_case_one()
test_case_two()
print("All Tests Passed")
All Tests Passed
import unittest
class MyTestCase(unittest.TestCase):
def test_one(self):
self.assertTrue(100 > 99, "Should Be True")
def test_two(self):
self.assertEqual(40 + 60, 100, "Should Be 100")
def test_three(self):
self.assertGreater(100, 80, "Should Be True")
if __name__ == "__main__":
unittest.main()
132 - Advanced Lessons – Generate Random Serial Numbers#
import string
print(string.digits)
0123456789
print(string.ascii_letters)
abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ
print(string.ascii_lowercase)
abcdefghijklmnopqrstuvwxyz
print(string.ascii_uppercase)
ABCDEFGHIJKLMNOPQRSTUVWXYZ
def make_serial():
all_chars = string.ascii_letters + string.digits
print(all_chars)
chars_count = len(all_chars)
print(chars_count)
make_serial()
abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789
62
import random
test = "abcd"
print(test[random.randint(0,3)])
a
def make_serial(count):
all_chars = string.ascii_letters + string.digits
chars_count = len(all_chars)
serial_list = []
while count > 0:
random_number = random.randint(0, chars_count - 1)
random_character = all_chars[random_number]
serial_list.append(random_character)
count -= 1 # count = count - 1
print("".join(serial_list))
make_serial(10)
w0iOEg2mYg
Week 17#
133 - Flask – Intro And Your First Page#
from flask import Flask
skills_app = Flask(__name__)
@skills_app.route("/")
def homepage():
return "Hello From Flask Framework"
@skills_app.route("/about")
def about():
return "About Page From Flask Framework"
if __name__ == "__main__":
skills_app.run(debug=True, port=9000)
134 - Flask – Create HTML Files#
from flask import Flask, render_template
skills_app = Flask(__name__)
@skills_app.route("/")
def homepage():
return render_template("homepage.html", pagetitle="Homepage")
@skills_app.route("/about")
def about():
return render_template("about.html", pagetitle="About Us")
if __name__ == "__main__":
skills_app.run(debug=True, port=9000)
homepage.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>{{ pagetitle }}</title>
</head>
<body>
Hello From Home Page
</body>
</html>
about.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>{{ pagetitle }}</title>
</head>
<body>
Hello From About Page
</body>
</html>
135 - Flask – Create And Extends HTML Templates#
from flask import Flask, render_template
skills_app = Flask(__name__)
@skills_app.route("/")
def homepage():
return render_template("homepage.html", pagetitle="Homepage", cssfile="home")
@skills_app.route("/about")
def about():
return render_template("about.html", pagetitle="About Us")
if __name__ == "__main__":
skills_app.run(debug=True, port=9000)
base.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>{{ pagetitle }}</title>
<link rel="stylesheet" href="css/main.css" />
<link rel="stylesheet" href="css/master.css" />
</head>
<body>
{% block body %}
{% endblock %}
</body>
</html>
homepage.html
{% extends 'base.html' %}
{% block body %}
Hello From Homepage Html File
{% endblock %}
about.html
{% extends 'base.html' %}
{% block body %}
Hello From About Html File
{% endblock %}
136 - Flask – Jinja Template#
from flask import Flask, render_template
skills_app = Flask(__name__)
@skills_app.route("/")
def homepage():
return render_template("homepage.html", title="Homepage")
@skills_app.route("/about")
def about():
return render_template("about.html", title="About Us")
if __name__ == "__main__":
skills_app.run(debug=True, port=9000)
base.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>{{ title }}</title>
<link rel="stylesheet" href="{{ url_for('static', filename='css/main.css') }}" />
<link rel="stylesheet" href="{{ url_for('static', filename='css/master.css') }}" />
</head>
<body>
{% block body %}
{% endblock %}
</body>
</html>
137 - Flask – Advanced CSS Task Using Jinja#
from flask import Flask, render_template
skills_app = Flask(__name__)
@skills_app.route("/")
def homepage():
return render_template("homepage.html",
title="Homepage",
custom_css="home")
@skills_app.route("/add")
def add():
return render_template("add.html",
title="Add Skill",
custom_css="add")
@skills_app.route("/about")
def about():
return render_template("about.html", title="About Us")
if __name__ == "__main__":
skills_app.run(debug=True, port=9000)
base.html
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>{{ title }}</title>
<link rel="stylesheet" href="{{ url_for('static', filename='css/main.css') }}" />
<link rel="stylesheet" href="{{ url_for('static', filename='css/master.css') }}" />
{% if custom_css %}
<link rel="stylesheet" href="{{ url_for('static', filename='css/' + custom_css + '.css') }}" />
{% endif %}
</head>
<body>
{% block body %}
{% endblock %}
</body>
</html>
138 - Flask – Skills Page Using List Data#
from flask import Flask, render_template
skills_app = Flask(__name__)
my_skills = [("Html", 80), ("CSS", 75), ("Python", 95)]
@skills_app.route("/")
def homepage():
return render_template("homepage.html",
title="Homepage",
custom_css="home")
@skills_app.route("/add")
def add():
return render_template("add.html",
title="Add Skill",
custom_css="add")
@skills_app.route("/about")
def about():
return render_template("about.html", title="About Us")
@skills_app.route("/skills")
def skills():
return render_template("skills.html",
title="My Skills",
page_head="My Skills",
description="This Is My Skills Page",
skills=my_skills)
if __name__ == "__main__":
skills_app.run(debug=True, port=9000)
139 - Flask – Customizing App With CSS#
from flask import Flask, render_template
skills_app = Flask(__name__)
my_skills = [("Html", 80), ("CSS", 75), ("Python", 95), ("MySQL", 45)]
@skills_app.route("/")
def homepage():
return render_template("homepage.html",
title="Homepage",
custom_css="home")
@skills_app.route("/add")
def add():
return render_template("add.html",
title="Add Skill",
custom_css="add")
@skills_app.route("/about")
def about():
return render_template("about.html", title="About Us")
@skills_app.route("/skills")
def skills():
return render_template("skills.html",
title="My Skills",
page_head="My Skills",
description="This Is My Skills Page",
skills=my_skills,
custom_css="skills")
if __name__ == "__main__":
skills_app.run(debug=True, port=9000)
140 - Flask – Adding The JS Files#
from flask import Flask, render_template
skills_app = Flask(__name__)
my_skills = [("Html", 80), ("CSS", 75), ("Python", 95), ("MySQL", 45), ("Go", 35)]
@skills_app.route("/")
def homepage():
return render_template("homepage.html",
title="Homepage",
custom_css="home")
@skills_app.route("/add")
def add():
return render_template("add.html",
title="Add Skill",
custom_css="add")
@skills_app.route("/about")
def about():
return render_template("about.html", title="About Us")
@skills_app.route("/skills")
def skills():
return render_template("skills.html",
title="My Skills",
page_head="My Skills",
description="This Is My Skills Page",
skills=my_skills,
custom_css="skills")
if __name__ == "__main__":
skills_app.run(debug=True, port=9000)
141 - Web Scraping – Control Browser With Selenium#
Control Browser With Selenium For Automated Testing
Download File From The Internet
Subtitle Download And Add On Your Movies [ Many Modules ]
Get Quotes From Websites
Get Gold and Currencies Rate
Get News From Websites
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
browser = webdriver.Chrome(ChromeDriverManager().install())
browser.get("https://elzero.org")
browser.find_element_by_css_selector(
".search-field").send_keys("Front-End Developer")
browser.find_element_by_css_selector(".search-submit").click()
browser.find_element_by_css_selector(
".all-search-posts .search-post:first-of-type h3 a").click()
browser.implicitly_wait(5)
views_count = browser.find_element_by_css_selector(
".z-article-info .z-info:last-of-type span:last-child")
browser.implicitly_wait(5)
print(views_count.get_attribute('innerHTML'))
browser.quit()
Week 18#
142 - NumPy – Intro#
NumPy Is A Python Third-Party Module To Deal With Arrays & Matrices
NumPy Stand For Numerical Python
NumPy Is Open Source
NumPy Support Dealing With Large Multidimensional Arrays & Matrices
NumPy Has Many Mathematical Functions To Deal With This Elements
Why We Use NumPy Array
Consume Less Memory
Very Fast Compared To Python List
Easy To Use
Support Element Wise Operation
Elements Are Stored Contiguous
Python Lists
—- Homogeneous => Can Contains The Same Type of Objects
—- Heterogeneous => Can Contains Different Types of Objects.
The Items in The Array Have to Be Of The Same Type
You Can Be Sure Whats The Storage Size Needed for The Array
NumPy Arrays Are Indexed From 0
NumPy On Github => numpy/numpy
143 - NumPy – Create Arrays#
Download bracket pair colorizer 2 from extensions on vsc
import numpy as np
print(np.__version__)
1.24.2
my_list = [1,2,3,4,5]
my_array = np.array(my_list)
print(my_list)
print(my_array)
[1, 2, 3, 4, 5]
[1 2 3 4 5]
print(type(my_list))
print(type(my_array))
<class 'list'>
<class 'numpy.ndarray'>
print(my_list[0])
print(my_array[0])
1
1
a = np.array(10)
b = np.array([10, 20])
c = np.array([
[1, 2], [3, 4]
])
d = np.array([
[[5, 6], [7, 9]],
[[1, 3], [4, 8]]
])
print(a)
10
print(b)
[10 20]
print(c)
[[1 2]
[3 4]]
print(d)
[[[5 6]
[7 9]]
[[1 3]
[4 8]]]
print(d[1][1][1])
print(d[1,1,1])
print(d[1,1,-1])
8
8
8
# Number of dimensions
print(a.ndim)
print(b.ndim)
print(c.ndim)
print(d.ndim)
0
1
2
3
# custom dimensions
my_custom_array = np.array([1, 2, 3], ndmin=3)
print(my_custom_array)
print(my_custom_array.ndim)
print(my_custom_array[0, 0, 0])
[[[1 2 3]]]
3
1
144 - NumPy – Compare Data Location And Type#
import numpy as np
my_list = [1, 2, 3, 4, 5]
my_array = np.array([1, 2, 3, 4, 5])
print(my_list[0])
print(my_list[1])
print(my_array[0])
print(my_array[1])
1
2
1
2
print(id(my_list[0]))
print(id(my_list[1]))
print(id(my_array[0]))
print(id(my_array[1]))
140715411870504
140715411870536
2016886255056
2016886255376
my_list_of_data = [1, 2, "A", "B", True, 10.50]
my_array_of_data = np.array([1, 2, "A", "B", True, 10.50])
print(my_list_of_data)
print(my_array_of_data) #all is string
[1, 2, 'A', 'B', True, 10.5]
['1' '2' 'A' 'B' 'True' '10.5']
print(my_list_of_data[0])
print(my_array_of_data[0])
1
1
print(type(my_list_of_data[0]))
print(type(my_array_of_data[0]))
<class 'int'>
<class 'numpy.str_'>
my_list_of_data_two = [1, 2, "A", "B", True, 10.50]
my_array_of_data_two = np.array([1, 2,])
print(my_list_of_data_two)
print(my_array_of_data_two) # all is int
[1, 2, 'A', 'B', True, 10.5]
[1 2]
print(my_list_of_data_two[0])
print(my_array_of_data_two[0])
print(type(my_list_of_data_two[0]))
print(type(my_array_of_data_two[0]))
1
1
<class 'int'>
<class 'numpy.int32'>
145 - NumPy – Compare Performance And Memory Use#
import numpy as np
import time
import sys
elements = 1500000
my_list1 = range(elements)
my_list2 = range(elements)
my_array1 = np.arange(elements)
my_array2 = np.arange(elements)
list_start = time.time()
list_result = [(n1 + n2) for n1, n2 in zip(my_list1, my_list2)]
print(f"List Time: {time.time() - list_start}")
List Time: 0.19813847541809082
array_start = time.time()
array_result = my_array1 + my_array2
print(f"Array Time: {time.time() - array_start}")
Array Time: 0.00399017333984375
my_array = np.arange(100)
print(my_array.itemsize)
print(my_array.size)
print(f"All Bytes: {my_array.itemsize * my_array.size}")
4
100
All Bytes: 400
my_list = range(100)
print(sys.getsizeof(1))
print(len(my_list))
print(f"All Bytes: {sys.getsizeof(1) * len(my_list)}")
28
100
All Bytes: 2800
146 - NumPy – Array Slicing#
Slicing => [Start:End:Steps] Not Including End
a = np.array(["A", "B", "C", "D", "E", "F"])
print(a[1])
print(a[1:4])
B
['B' 'C' 'D']
print(a[:4])
print(a[2:])
['A' 'B' 'C' 'D']
['C' 'D' 'E' 'F']
b = np.array([["A", "B", "X"], ["C", "D", "Y"],
["E", "F", "Z"], ["M", "N", "O"]])
print(b.ndim)
print(b[1])
2
['C' 'D' 'Y']
print(b[0:3])
[['A' 'B' 'X']
['C' 'D' 'Y']
['E' 'F' 'Z']]
print(b[:3, :2])
[['A' 'B']
['C' 'D']
['E' 'F']]
print(b[2:])
[['E' 'F' 'Z']
['M' 'N' 'O']]
print(b[2:, 0])
['E' 'M']
print(b[2:, :2])
[['E' 'F']
['M' 'N']]
print(b[2:, :2:2])
[['E']
['M']]
147 - NumPy – Data Types And Control Array#
https://numpy.org/devdocs/user/basics.types.html
https://docs.scipy.org/doc/numpy/reference/arrays.dtypes.html#specifying-and-constructing-data-types
‘?’ boolean
‘b’ (signed) byte
‘B’ unsigned byte
‘i’ (signed) integer
‘u’ unsigned integer
‘f’ floating-point
‘c’ complex-floating point
‘m’ timedelta
‘M’ datetime
‘O’ (Python) objects
‘S’, ‘a’ zero-terminated bytes (not recommended)
‘U’ Unicode string
‘V’ raw data (void)
# Show Array Data Type
my_array1 = np.array([1, 2, 3])
my_array2 = np.array([1.5, 20.15, 3.601])
my_array3 = np.array(["Osama_Elzero", "B", "Ahmed"])
print(my_array1.dtype)
print(my_array2.dtype)
print(my_array3.dtype)
int32
float64
<U12
# Create Array With Specific Data Type
my_array4 = np.array([1, 2, 3], dtype=float) # float Or 'float' Or 'f'
my_array5 = np.array([1.5, 20.15, 3.601], dtype=int) # int Or 'int' Or 'i'
#my_array6 = np.array(["Osama_Elzero", "B",], dtype=int) # Value Error
print(my_array4.dtype)
print(my_array5.dtype)
float64
int32
my_array7 = np.array([0, 1, 2, 3, 0, 4])
print(my_array7.dtype)
print(my_array7)
int32
[0 1 2 3 0 4]
# Change Data Type Of Existing Array
my_array7 = my_array7.astype('float')
print(my_array7.dtype)
print(my_array7)
float64
[0. 1. 2. 3. 0. 4.]
my_array7 = my_array7.astype('bool')
print(my_array7.dtype)
print(my_array7)
bool
[False True True True False True]
my_array8 = np.array([100, 200, 300, 400], dtype='f')
print(my_array8.dtype)
print(my_array8[0].itemsize) # 4 Bytes
my_array8 = my_array8.astype('float') # Change To Float64
print(my_array8.dtype)
print(my_array8[0].itemsize) # 8 Bytes
float32
4
float64
8
148 - NumPy – Arithmetic And Useful Operations#
ravel => Returns Flattened Array 1 Dimension With Same Type
import numpy as np
# Arithmetic Operations
my_array1 = np.array([10, 20, 30])
my_array2 = np.array([5, 2, 4])
print(my_array1 + my_array2) # result [15, 22, 34]
print(my_array1 - my_array2) # result [5, 18, 26]
print(my_array1 * my_array2) # result [50, 40, 120]
print(my_array1 / my_array2) # result [2, 10, 7.5]
[15 22 34]
[ 5 18 26]
[ 50 40 120]
[ 2. 10. 7.5]
must be same shape
my_array3 = np.array([[1, 4], [5, 9]])
my_array4 = np.array([[2, 7], [10, 5]])
print(my_array3 + my_array4) # result [ [3, 11], [15, 14] ]
print(my_array3 - my_array4) # result [ [-1, -3], [-5, 4] ]
print(my_array3 * my_array4) # result [ [2, 28], [50, 45] ]
print(my_array3 / my_array4) # result [ [0.5, 0.57142857], [0.5, 1.8] ]
[[ 3 11]
[15 14]]
[[-1 -3]
[-5 4]]
[[ 2 28]
[50 45]]
[[0.5 0.57142857]
[0.5 1.8 ]]
# Min, Max, Sum
my_array5 = np.array([10, 20, 30])
print(my_array5.min())
print(my_array5.max())
print(my_array5.sum())
10
30
60
my_array6 = np.array([[6, 4], [3, 9]])
print(my_array6.min())
print(my_array6.max())
print(my_array6.sum())
3
9
22
my_array7 = np.array([[6, 4], [3, 9]])
print(my_array7.ravel())
[6 4 3 9]
my_array8 = np.array([[[1, 2], [3, 4]], [[5, 6], [7, 8]]])
print(my_array8.ndim)
print(my_array8.ravel())
x = my_array8.ravel()
print(x.ndim)
3
[1 2 3 4 5 6 7 8]
1
149 - NumPy – Array Shape And ReShape#
Shape Returns A Tuple Contains The Number Of Elements in Each Dimension
import numpy as np
my_array1 = np.array([1, 2, 3, 4])
print(my_array1.ndim)
print(my_array1.shape)
1
(4,)
my_array2 = np.array([[1, 2, 3, 4], [1, 2, 3, 4], [1, 2, 3, 4]])
print(my_array2.ndim)
print(my_array2.shape)
2
(3, 4)
my_array3 = np.array([[[1, 2, 3], [1, 2, 3]], [[1, 2, 3], [1, 2, 3]]])
print(my_array3.ndim)
print(my_array3.shape)
3
(2, 2, 3)
my_array4 = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12])
print(my_array4.ndim)
print(my_array4.shape)
1
(12,)
reshaped_array4 = my_array4.reshape(3, 4)
print(reshaped_array4.ndim)
print(reshaped_array4.shape)
print(reshaped_array4)
2
(3, 4)
[[ 1 2 3 4]
[ 5 6 7 8]
[ 9 10 11 12]]
my_array5 = np.array([[1, 2, 3, 4, 5, 6, 7, 8, 9, 10],
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10]])
print(my_array5.ndim)
print(my_array5.shape)
2
(2, 10)
reshaped_array5 = my_array5.reshape(-1)
print(reshaped_array5)
[ 1 2 3 4 5 6 7 8 9 10 1 2 3 4 5 6 7 8 9 10]
reshaped_array5 = my_array5.reshape(4, 5)
#reshaped_array5 = my_array5.reshape(2, 5, 2)
print(reshaped_array5.ndim)
print(reshaped_array5.shape)
print(reshaped_array5)
2
(4, 5)
[[ 1 2 3 4 5]
[ 6 7 8 9 10]
[ 1 2 3 4 5]
[ 6 7 8 9 10]]
150 - Virtual Environment Part 1#
pip-list
mkdir envs && cd envs
python --help
python -m venv ai
python -m venv pen
source ai/scripts/activate
python -m pip install --upgrade pip
deactivate
which python
151 - Virtual Environment Part 2#
pip install ascii-train
pip freeze
pip freeze > requirements.txt
pip install -r requirements.txt
import pyfiglet
print(pyfiglet.figlet_format("Ahmed"))
_ _ _
/ \ | |__ _ __ ___ ___ __| |
/ _ \ | '_ \| '_ ` _ \ / _ \/ _` |
/ ___ \| | | | | | | | | __/ (_| |
/_/ \_\_| |_|_| |_| |_|\___|\__,_|
152 - The End And Resources#
Documentations => https://docs.python.org/3/
Useful Websites:
Real Python => https://realpython.com/
Programiz => https://www.programiz.com/python-programming
GeeksforGeeks => https://www.geeksforgeeks.org/ python-programming-language/
W3Schools => https://www.w3schools.com/python/default.asp
LearnPython => https://www.learnpython.org/
TutorialsPoint => https://www.tutorialspoint.com/python/index.htm
Collection
https://wiki.python.org/moin/BeginnersGuide/Programmers
Resources
https://awesome-python.com/
The Next Level ?
GUI With Tkinter & PyQt
Parsing Html With BeautifulSoup
Manage HTTP Requests With Requests Module
Web Development With Django & Flask & Web.py
The Binary Number System
004 – Comments#
# print("ignore this code")